Patient Summary module
Patient Summary page
This is a root page for information about current Patient. Patient summary page has two view types:
- patient summary roll
- patient summary table
Patient Summary Table
Patient Summary roll includes following elements:
- Breadcrumbs
- Settings modal window
- Patient anthropometric information block
- Block with four latest items of Problems, Medications and Allergies
- Lab results block
- Events timeline block
- Vitals chart block
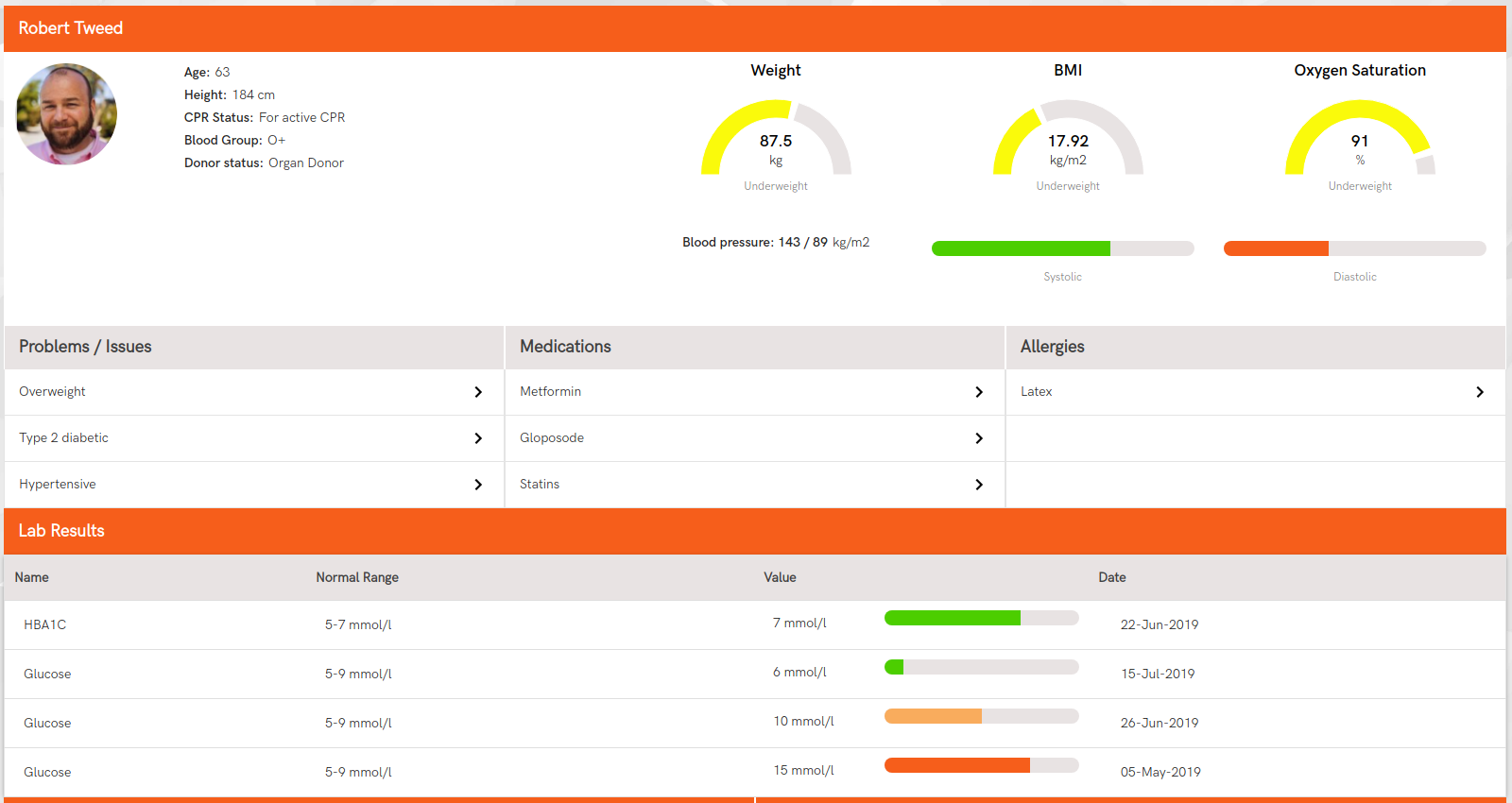
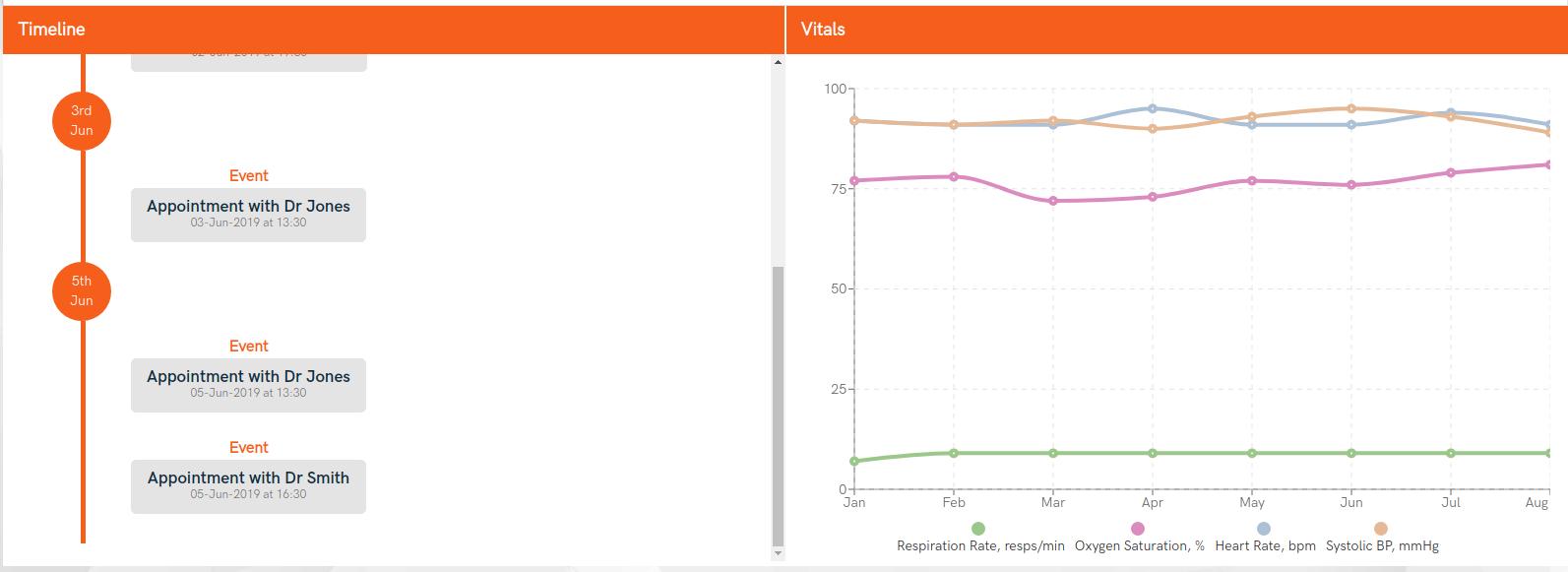
Patient Summary Table
Patient Summary table includes following elements:
- Breadcrumbs
- Settings modal window
- Synopsis grid
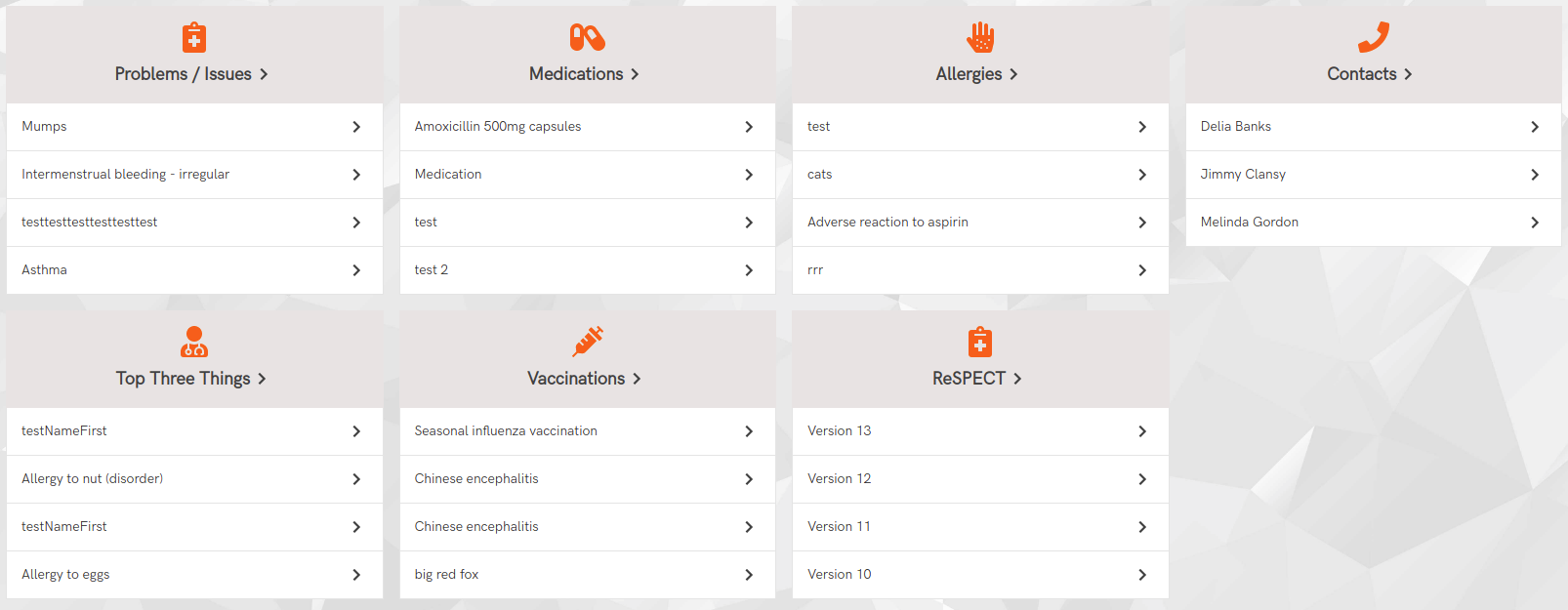
Synopsis grid contains panels with synopsis for plugins Allergies, Contacts, Medications, Problems, Top Three Things, Vaccinations and ReSPECT. Synopsis is a list of the last four issues for each plugin. Each synopsis returns by the separate API-request.
Click on any of synopsis items redirects user to details page for it. Click on the panel title redirects user to the list for the selected plugin.
Component structure
import React, { Component } from "react"; import get from "lodash/get"; import { connect } from 'react-redux'; import { withStyles } from "@material-ui/core/styles"; import Grid from '@material-ui/core/Grid'; import DashboardCard from "../../common/DashboardCard"; import { synopsisAllergiesAction, synopsisContactsAction, synopsisMedicationsAction, synopsisProblemsAction } from "../../actions/synopsisActions"; import { synopsisData, getSynopsisProps } from "./config"; import SettingsDialog from "./SettingsDialog"; import Breadcrumbs from "../../common/Breadcrumbs"; import { themeCommonElements } from "../../../version/config/theme.config"; import { nonCoreSynopsisActions } from "../../../version/config/nonCoreSynopsis"; import { getSummaryContainerStyles } from "./functions"; const styles = theme => ({ ... ... ... }); class PatientSummaryInfo extends Component { componentDidMount() { if (localStorage.getItem('userId') && localStorage.getItem('username')) { this.props.getPatientSynopsis(); } } render() { const { classes, loading, showMode, showHeadings, location } = this.props; const breadcrumbsResource = [ { url: location.pathname, title: "Patient Summary", isActive: false } ]; const FeedsPanels = get(themeCommonElements, 'feedsPanels', false); const RespectPanel = get(themeCommonElements, 'respectPanel', false); return ( <Grid className={classes.container} > <Breadcrumbs resource={breadcrumbsResource} /> <SettingsDialog className={classes.settingsIcon} /> <Grid className={classes.summaryContainer} spacing={16} container> { synopsisData.map((item, key) => { return ( <DashboardCard key={key} showMode={showMode} showHeadings={showHeadings} id={item.id} title={item.title} list={item.list} loading={loading} items={get(this.props, item.list, [])} icon={item.icon} {...this.props} /> ); }) } { FeedsPanels && <FeedsPanels /> } { RespectPanel && <RespectPanel showMode={showMode} {...this.props} /> } </Grid> </Grid> ); } } const mapStateToProps = state => { const patientSummaryProps = { loading: state.custom.demographics.loading, showMode: state.custom.showMode.data, showHeadings: state.custom.showHeadings.data, }; const synopsisProps = getSynopsisProps(state); return Object.assign({}, patientSummaryProps, synopsisProps); }; const mapDispatchToProps = dispatch => { const coreSynopsisActions = [ synopsisAllergiesAction, synopsisContactsAction, synopsisProblemsAction, synopsisMedicationsAction, ]; const synopsisActions = coreSynopsisActions.concat(nonCoreSynopsisActions); return { getPatientSynopsis() { synopsisActions.map(item => { return dispatch(item.request()); }); } } }; export default connect(mapStateToProps, mapDispatchToProps)(withStyles(styles)(PatientSummaryInfo));
Patient Allergies synopsis
API URL
/api/patients/{patientId}/synopsis/allergies
GET data
{ heading: "allergies", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1560263418000, source: "ethercis", sourceId: "ethercis-11d2021c-f1a9-4258-800e-9e141b1521b4", text: "Adverse reaction to aspirin", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }
Patient Contacts synopsis
API URL
/api/patients/{patientId}/synopsis/contacts
GET data
{ heading: "contacts", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1560330131000, source: "ethercis", sourceId: "ethercis-996d182e-89bb-4a0c-9c0e-41f962cf34e5", text: "Jimmy Clansy", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }
Patient Medications synopsis
API URL
/api/patients/{patientId}/synopsis/medications
GET data
{ heading: "medications", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1560332128000, source: "ethercis", sourceId: "ethercis-f28f0c62-04f4-434c-8f80-f3e7cdcbd425", text: "Amoxicillin 500mg capsules", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }
Patient Problems/Issues synopsis
API URL
/api/patients/{patientId}/synopsis/problems
GET data
{ heading: "problems", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1560332128000, source: "ethercis", sourceId: "ethercis-f28f0c62-04f4-434c-8f80-f3e7cdcbd425", text: "Amoxicillin 500mg capsules", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }
Patient Vaccinations synopsis
API URL
/api/patients/{patientId}/synopsis/vaccinations
GET data
{ heading: "vaccinations", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1559650691000, source: "ethercis", sourceId: "ethercis-e57aaa81-18de-44a8-855a-d8bee1149f32", text: "Chinese encephalitis", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }
Patient Top Three Things synopsis
API URL
/api/patients/{patientId}/synopsis/top3Things
GET data
{ heading: "top3Things", synopsis: [ 0: {...}, 1: {...}, 2: { dateCreated: 1559911963000, source: "ethercis", sourceId: "ethercis-884829e1-ea10-42be-809d-b30afd998bc6", text: "Allergy to eggs", }, 3: {...}, ], token: "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...." }