Test Results module
Test Results
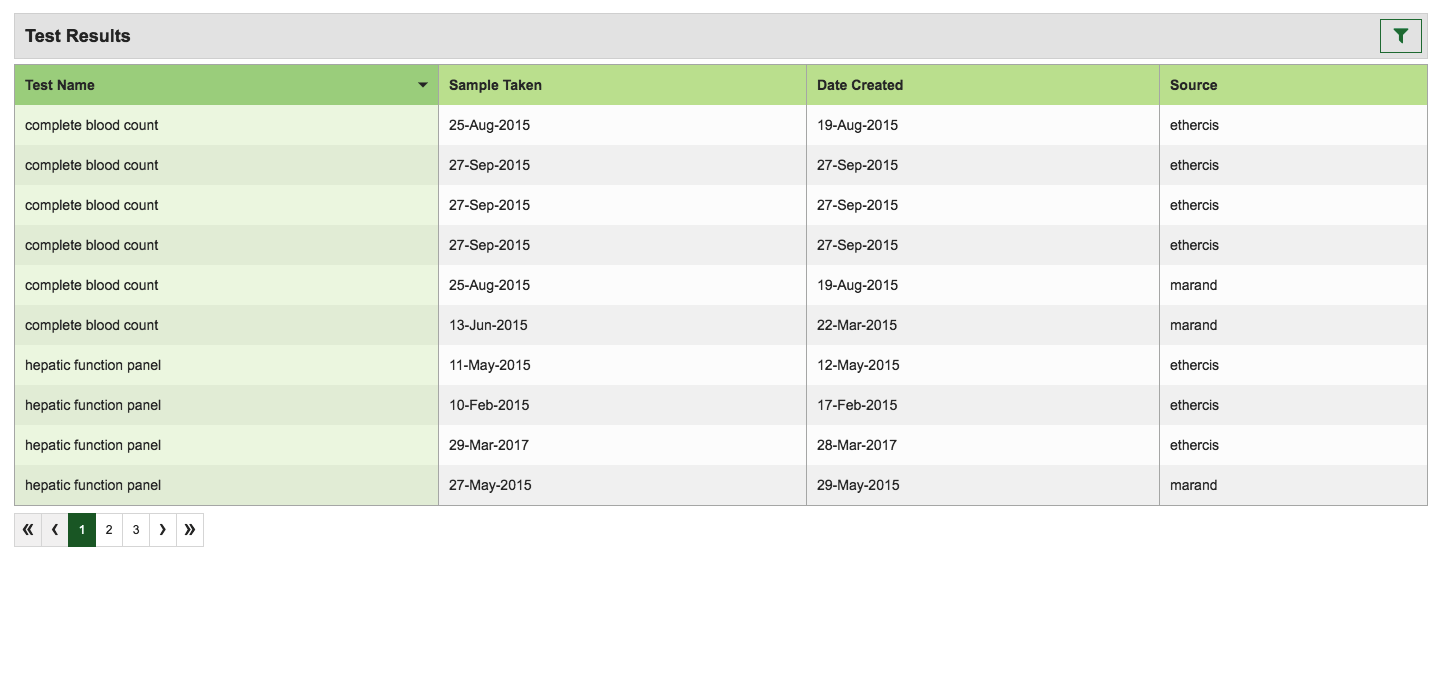
API URL
/api/patients/{patientId}/labresults
GET response
{ dateCreated:1427004662000 sampleTaken:1426889462518 source:"ethercis" sourceId:"810272ac-28e8-4928-b61b-79dcef4b4170" testName:"" }
Component structure
// import packages import React, { PureComponent } from 'react'; import { bindActionCreators } from 'redux'; import { connect } from 'react-redux'; import { lifecycle, compose } from 'recompose'; import PluginListHeader from '../../plugin-page-component/PluginListHeader'; import PluginMainPanel from '../../plugin-page-component/PluginMainPanel'; import { fetchPatientTestResultsRequest } from './ducks/fetch-patient-test-results.duck'; import { fetchPatientTestResultsDetailRequest } from './ducks/fetch-patient-test-results-detail.duck'; import { fetchPatientTestResultsOnMount } from '../../../utils/HOCs/fetch-patients.utils'; import { patientTestResultsSelector, patientTestResultsDetailSelector } from './selectors'; import { operationsOnCollection } from '../../../utils/plugin-helpers.utils'; import TestResultsDetail from './TestResultsDetail/TestResultsDetail'; // map dispatch to Properties const mapDispatchToProps = dispatch => ({ actions: bindActionCreators({ fetchPatientTestResultsRequest, fetchPatientTestResultsDetailRequest }, dispatch) }); // Higher-Order Components (HOC) for get some data @connect(patientTestResultsSelector, mapDispatchToProps) @connect(patientTestResultsDetailSelector, mapDispatchToProps) @compose(lifecycle(fetchPatientTestResultsOnMount)) export default class TestResults extends PureComponent { // React component // component template render() { return () } }
Test Results Detail
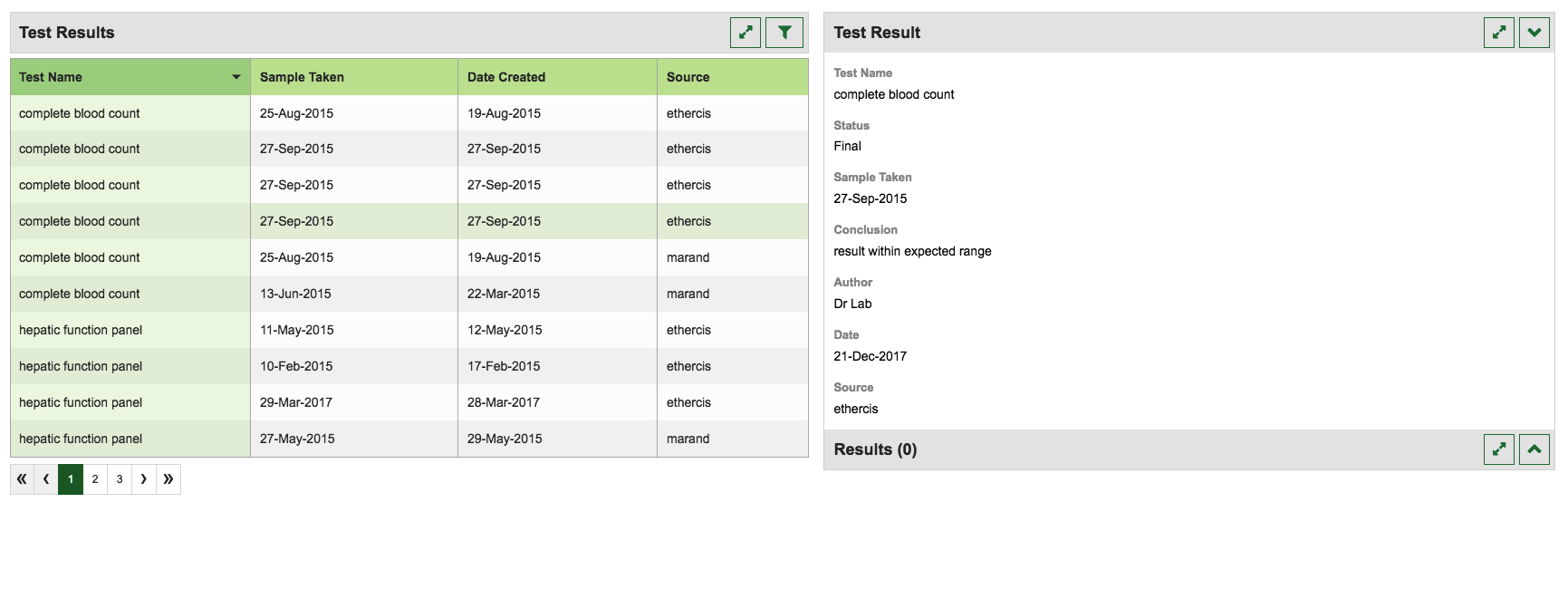
API URL
/api/patients/{patientId}/labresults/{sourceId}
GET response
{ author:"Dr Lab" conclusion:"" dateCreated:1437624662000 sampleTaken:1437513062518 source:"EtherCIS" sourceId:"b97e9fde-d994-4874-b671-8b1cd81b811c" status:"" testName:"" testResults:[{result: "", value: "", unit: "", normalRange: "", comment: ""}] }
Component structure
// import packages import React, { PureComponent } from 'react'; import PluginDetailPanel from '../../../plugin-page-component/PluginDetailPanel' import { valuesNames, valuesLabels } from '../forms.config'; export default class TestResultsDetail extends PureComponent { // React component // component template render() { return () } }
Test Results List Duck
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; // Actions names export const FETCH_PATIENT_TEST_RESULTS_REQUEST = 'FETCH_PATIENT_TEST_RESULTS_REQUEST'; export const FETCH_PATIENT_TEST_RESULTS_SUCCESS = 'FETCH_PATIENT_TEST_RESULTS_SUCCESS'; export const FETCH_PATIENT_TEST_RESULTS_FAILURE = 'FETCH_PATIENT_TEST_RESULTS_FAILURE'; // Actions export const fetchPatientTestResultsRequest = createAction(FETCH_PATIENT_TEST_RESULTS_REQUEST); export const fetchPatientTestResultsSuccess = createAction(FETCH_PATIENT_TEST_RESULTS_SUCCESS); export const fetchPatientTestResultsFailure = createAction(FETCH_PATIENT_TEST_RESULTS_FAILURE); // Epics for async actions export const fetchPatientTestResultsEpic = (action$, store) => {}; // reducer export default function reducer(patientsTestResults = {}, action) { switch (action.type) { case FETCH_PATIENT_TEST_RESULTS_SUCCESS: return _.set(action.payload.userId, action.payload.testResults, patientsTestResults); default: return patientsTestResults; } }
Test Results Detail Duck
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; // Actions names export const FETCH_PATIENT_TEST_RESULTS_DETAIL_REQUEST = 'FETCH_PATIENT_TEST_RESULTS_DETAIL_REQUEST'; export const FETCH_PATIENT_TEST_RESULTS_DETAIL_SUCCESS = 'FETCH_PATIENT_TEST_RESULTS_DETAIL_SUCCESS'; export const FETCH_PATIENT_TEST_RESULTS_DETAIL_FAILURE = 'FETCH_PATIENT_TEST_RESULTS_DETAIL_FAILURE'; // Actions export const fetchPatientTestResultsDetailRequest = createAction(FETCH_PATIENT_TEST_RESULTS_DETAIL_REQUEST); export const fetchPatientTestResultsDetailSuccess = createAction(FETCH_PATIENT_TEST_RESULTS_DETAIL_SUCCESS); export const fetchPatientTestResultsDetailFailure = createAction(FETCH_PATIENT_TEST_RESULTS_DETAIL_FAILURE); // Epics for async actions export const fetchPatientTestResultsDetailEpic = (action$, store) => {}; // reducer export default function reducer(testResultsDetail = {}, action) { switch (action.type) { case FETCH_PATIENT_TEST_RESULTS_DETAIL_SUCCESS: return _.set(action.payload.userId, action.payload.testResultsDetail, testResultsDetail); default: return testResultsDetail; } }