Clinical Statements module
Clinical Statements List
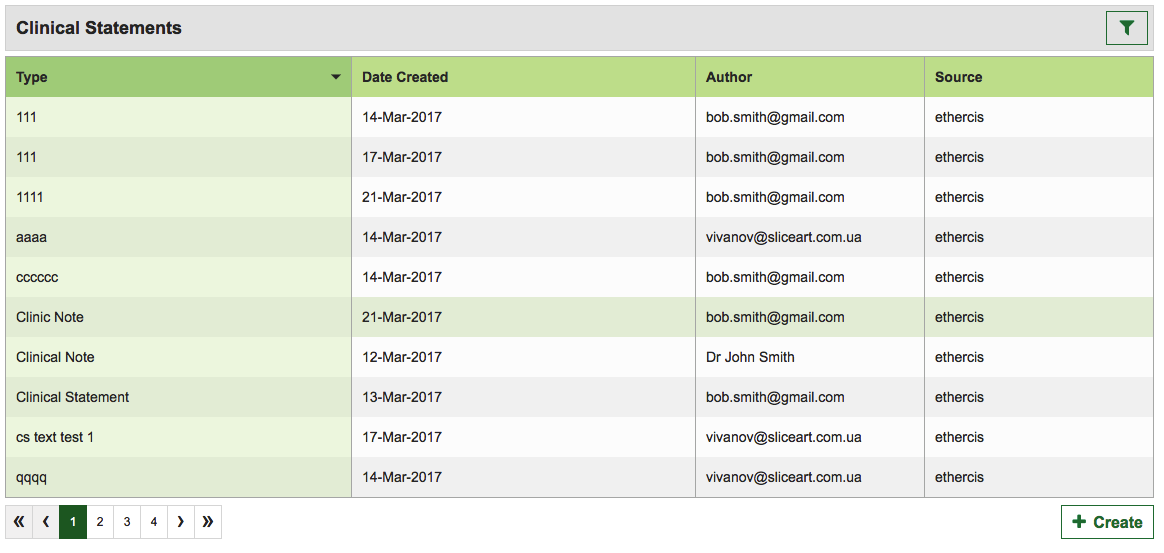
API URL
/api/patients/{patientId}/clinicalStatements
GET response
{ author:"bob.smith@gmail.com" dateCreated:1489655645844 source:"ethercis" sourceId:"0f86daea-9d25-4921-aa9c-b79b6478433d" type:"test ids" }
Component structure
//component template let templateClinicalstatementsList = require('./clinicalstatements-list.html'); //controller init class ClinicalstatementsListController { constructor($scope, $state, $stateParams, $ngRedux, clinicalstatementsActions, serviceRequests, usSpinnerService, serviceFormatted) { } //component init const ClinicalstatementsListComponent = { template: templateClinicalstatementsList, controller: ClinicalstatementsListController }; //inject services/modules to controller ClinicalstatementsListController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'clinicalstatementsActions', 'serviceRequests', 'usSpinnerService', 'serviceFormatted']; //es6 export for component export default ClinicalstatementsListComponent;
Clinical Statements Detail
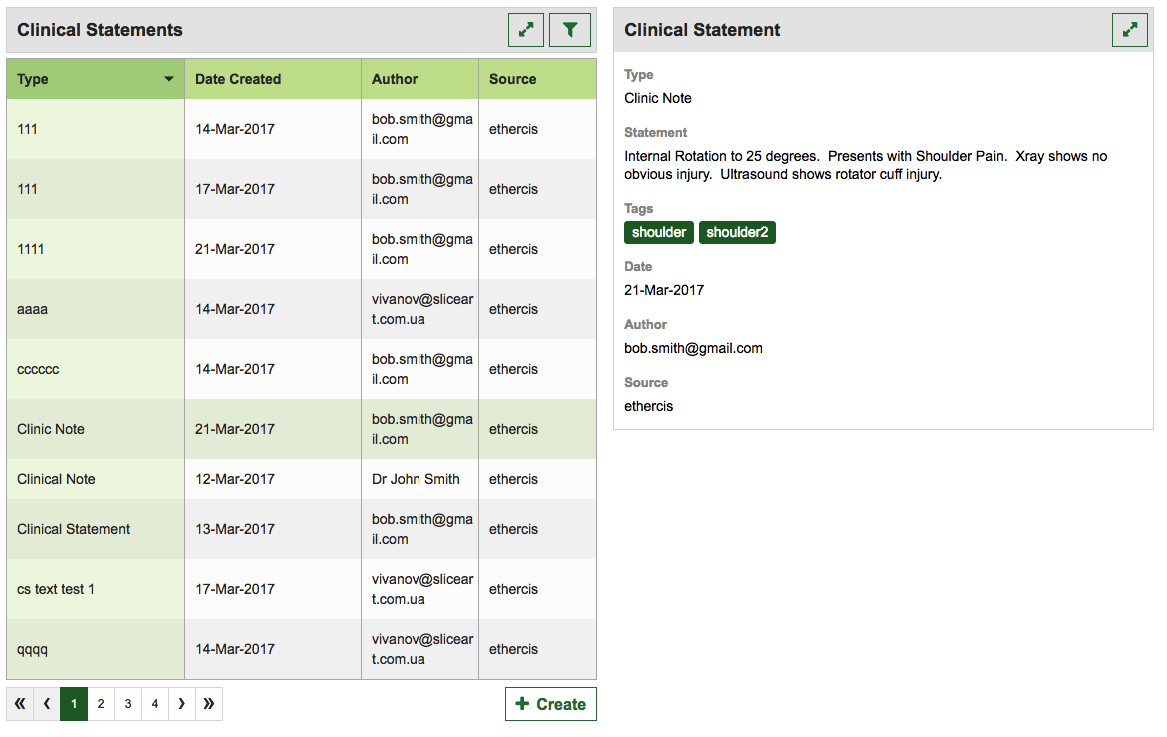
API URL
/api/patients/{patientId}/clinicalStatements/{sourceId}
GET response
{ author:"bob.smith@gmail.com" dateCreated:1489489481713 source:"ethercis" sourceId:"f546b8c0-97d6-489f-97e1-02622d721dc2" text:"The pain was medium at 5/10 in severity. " type:"aaaa" }
Component structure
//es6 import modules import * as helper from './clinicalstatements-helper'; //component template let templateClinicalstatementsDetail = require('./clinicalstatements-detail.html'); //controller init class ClinicalstatementsDetailController { constructor($scope, $state, $stateParams, $ngRedux, clinicalstatementsActions, serviceRequests, usSpinnerService) { } //component init const ClinicalstatementsDetailComponent = { template: templateClinicalstatementsDetail, controller: ClinicalstatementsDetailController }; //inject services/modules to controller ClinicalstatementsDetailController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'clinicalstatementsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default ClinicalstatementsDetailComponent;
Clinical Statements Create
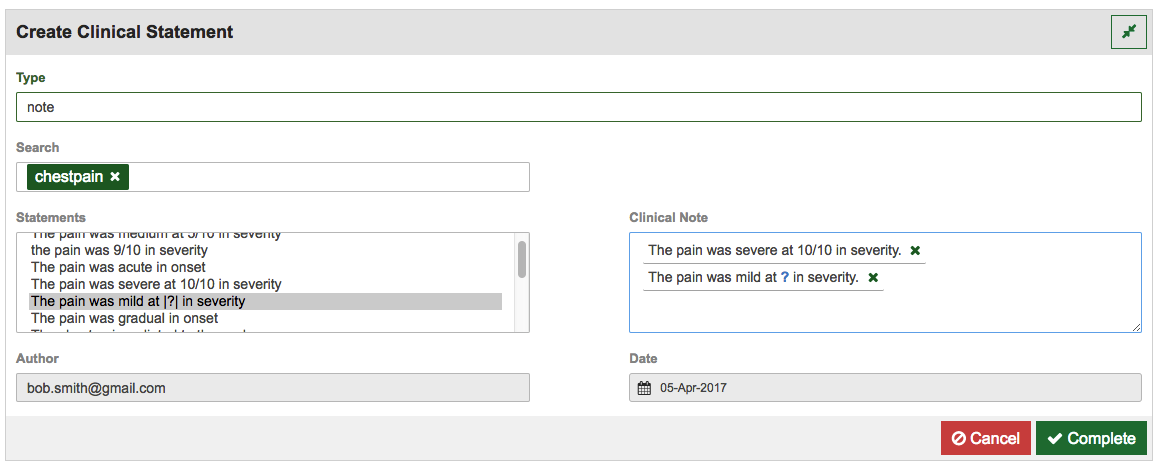
API URL
/api/patients/{patientId}/clinicalStatements
POST data
{
author:"bob.smith@gmail.com"
contentStore:{name: "ts", phrases: [{id: "6", tag: "chestpain"}]}
dateCreated:"2017-04-07T09:49:29.918Z"
text:'The pain was severe at 10/10 in severity. '
type:"test CS 33"
}
Component structure
//es6 import modules import * as helper from './clinicalstatements-helper'; //component template let templateClinicalstatementsCreate = require('./clinicalstatements-create.html'); let _ = require('underscore'); //controller init class ClinicalstatementsCreateController { constructor($scope, $state, $stateParams, $ngRedux, clinicalstatementsActions, usSpinnerService, serviceRequests, serviceFormatted) { } //component init const ClinicalstatementsCreateComponent = { template: templateClinicalstatementsCreate, controller: ClinicalstatementsCreateController }; //inject services/modules to controller ClinicalstatementsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'clinicalstatementsActions', 'usSpinnerService', 'serviceRequests', 'serviceFormatted']; //es6 export for component export default ClinicalstatementsCreateComponent;
Clinical Statements Actions
Component structure
//es6 import modules import {bindActionCreators} from 'redux'; import * as types from '../../../constants/ActionTypes'; //es6 export function export function all(patientId) { return { types: [types.CLINICALSTATEMENTS, types.CLINICALSTATEMENTS_SUCCESS, types.CLINICALSTATEMENTS_ERROR], shouldCallAPI: (state) => !state.clinicalstatements.response, config: { method: 'get', url: '/api/patients/' + patientId + '/clinicalStatements' }, meta: { timestamp: Date.now() } }; }
Clinical Statements Reducer
Component structure
//es6 import modules import * as types from '../../../constants/ActionTypes'; const INITIAL_STATE = { isFetching: false, error: false, data: null, dataGet: null, dataCreate: null, dataUpdate: null }; //es6 export function export default function clinicalstatements(state = INITIAL_STATE, action) { const {payload} = action; //redux action for Clinical statements requests var actions = { [types.CLINICALSTATEMENTS]: (state) => { state.dataCreate = null; state.dataUpdate = null; return Object.assign({}, state, { isFetching: true, error: false }); } }