Vitals module
Vitals List
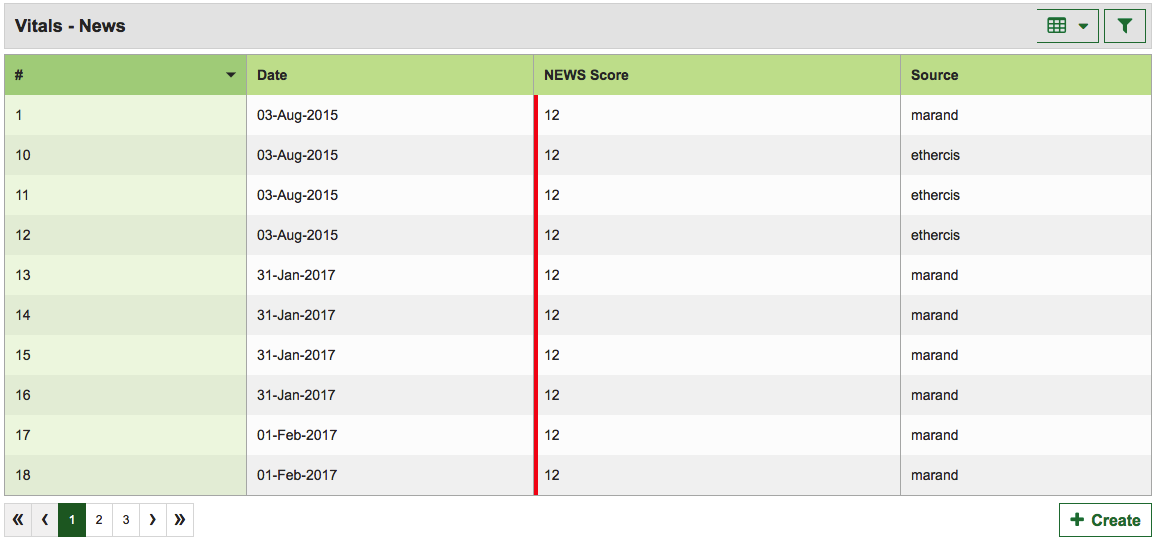
API URL
/api/patients/{patientId}/vitalsigns
GET response
{ author:"Dr Tony Shannon" dateCreate:1438572182000 diastolicBP:"64.0" heartRate:"45.0" levelOfConsciousness:"Alert" newsScore:12 oxygenSaturation:"97.0" oxygenSupplemental:"true" respirationRate:"23.0" source:"ethercis" sourceId:"27ee5e25-4c32-46d2-b45a-f74149d72030" systolicBP:"92.0" temperature:35.4 }
Component structure
//component template let templateVitalsList = require('./vitals-list.html'); //controller init class VitalsListController { constructor($scope, $state, $stateParams, $ngRedux, vitalsActions, serviceRequests, usSpinnerService, $window, $timeout, serviceVitalsSigns, serviceFormatted) { } //component init const VitalsListComponent = { template: templateVitalsList, controller: VitalsListController }; //inject services/modules to controller VitalsListController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'vitalsActions', 'serviceRequests', 'usSpinnerService', '$window', '$timeout', 'serviceVitalsSigns', 'serviceFormatted']; //es6 export for component export default VitalsListComponent;
Vitals Detail
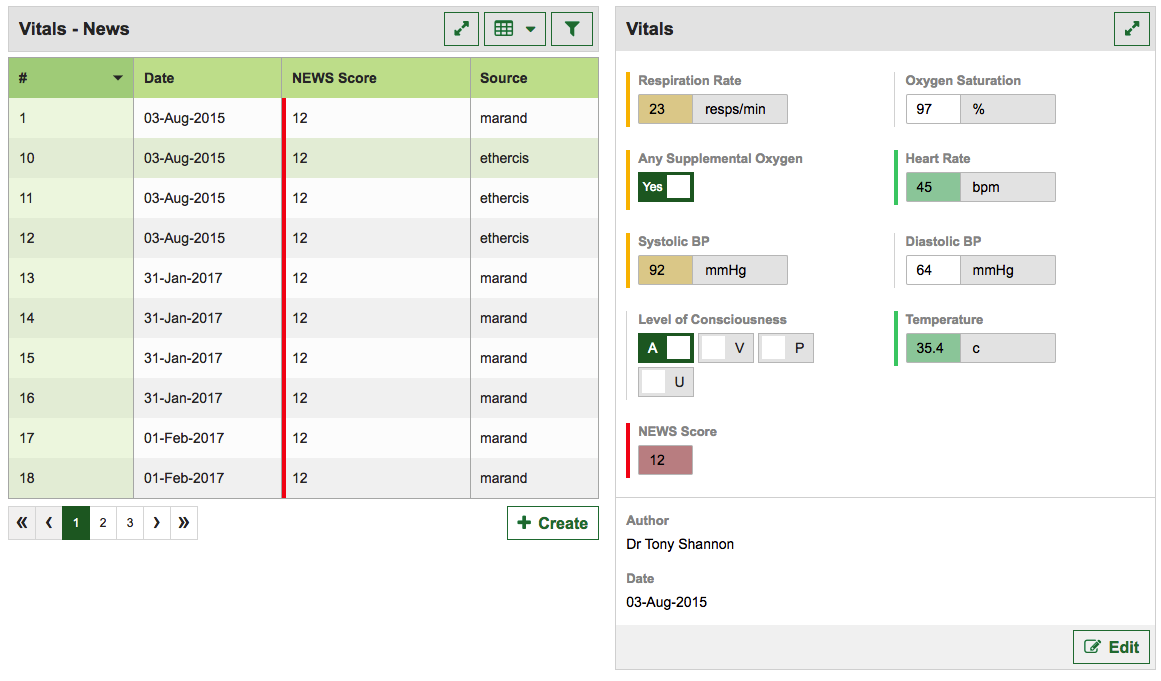
API URL
/api/patients/{patientId}/vitalsigns/{sourceId}
GET response
{ author:"Dr Tony Shannon" dateCreate:1438553462518 diastolicBP:60 heartRate:45 levelOfConsciousness:"Alert" newsScore:12 oxygenSaturation:97 oxygenSupplemental:"false" respirationRate:25 source:"Marand" sourceId:"2f4dff89-a41b-465b-85b6-b81d8a59e7a0" systolicBP:90 temperature:35.4 }
Component structure
//component template let templateVitalsDetail = require('./vitals-detail.html'); //controller init class VitalsDetailController { constructor($scope, $state, $stateParams, $ngRedux, patientsActions, vitalsActions, serviceRequests, usSpinnerService, serviceVitalsSigns) { } //component init const VitalsDetailComponent = { template: templateVitalsDetail, controller: VitalsDetailController }; //inject services/modules to controller VitalsDetailController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'patientsActions', 'vitalsActions', 'serviceRequests', 'usSpinnerService', 'serviceVitalsSigns']; //es6 export for component export default VitalsDetailComponent;
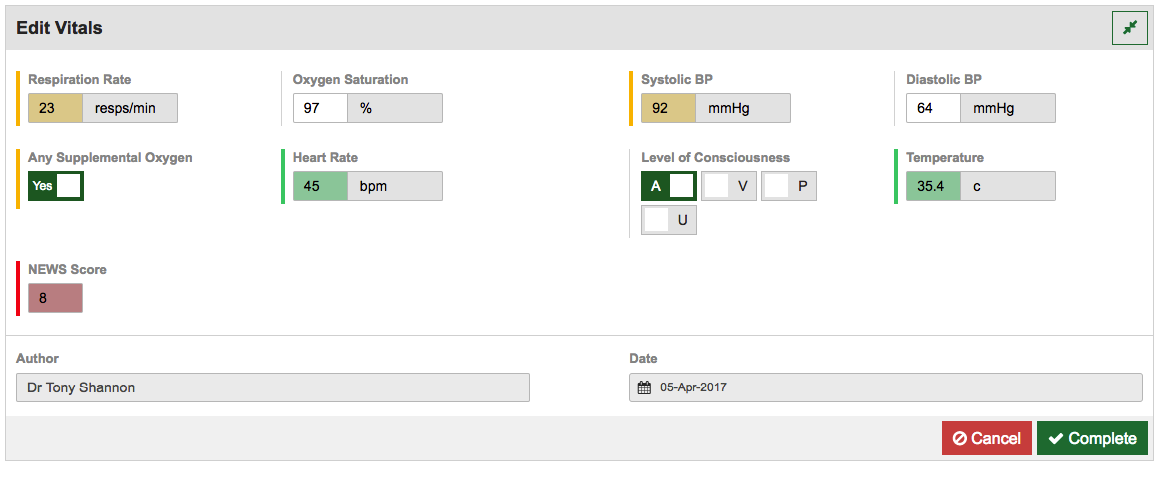
API URL
/api/patients/{patientId}/vitalsigns
GET response
{ author:"Dr Tony Shannon" dateCreate:1491571062000 diastolicBP:60 heartRate:45 levelOfConsciousness:"Pain" newsScore:11 oxygenSaturation:97 oxygenSupplemental:false respirationRate:25 source:"Marand" sourceId:"2f4dff89-a41b-465b-85b6-b81d8a59e7a0" systolicBP:90 temperature:35.4 }
Vitals Create
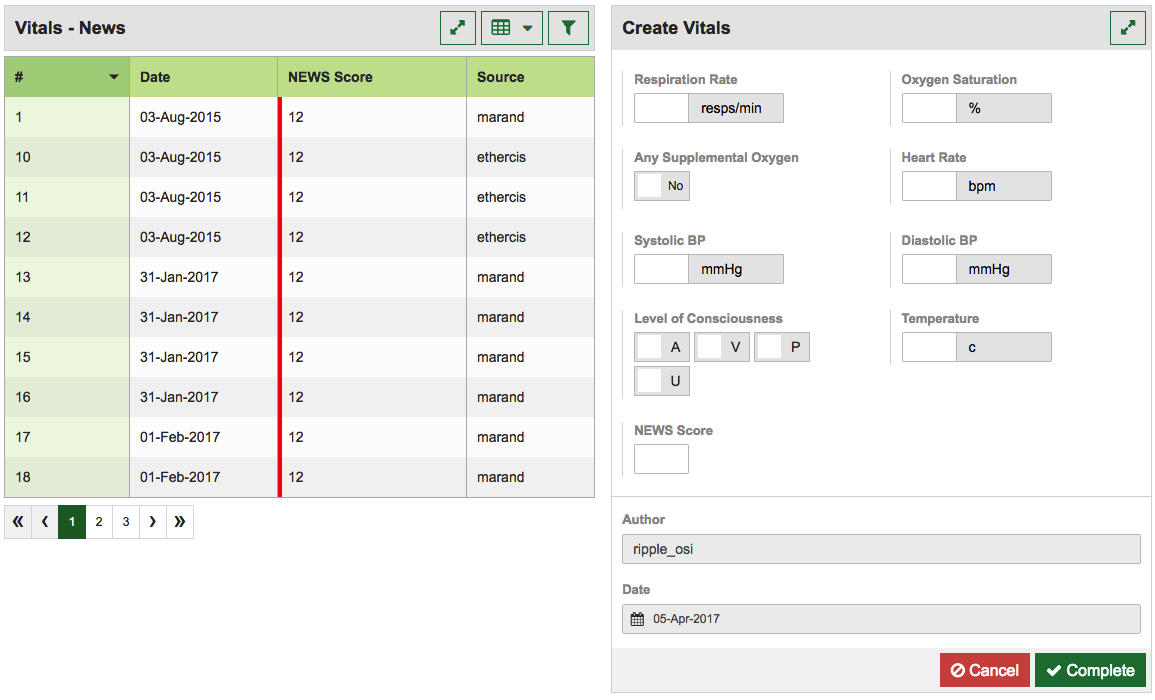
API URL
/api/patients/{patientId}/vitalsigns
POST response
{ author:"ripple_osi" dateCreate:1491571176000 diastolicBP:"77" heartRate:"55" levelOfConsciousness:"Verbal" newsScore:16 oxygenSaturation:"33" oxygenSupplemental:true respirationRate:"33" systolicBP:"88" temperature:"88" }
Component structure
//component template let templateVitalsCreate = require('./vitals-create.html'); //controller init class VitalsCreateController { constructor($scope, $state, $stateParams, $ngRedux, patientsActions, vitalsActions, serviceRequests, serviceVitalsSigns) { } //component init const VitalsCreateComponent = { template: templateVitalsCreate, controller: VitalsCreateController }; //inject services/modules to controller VitalsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'patientsActions', 'vitalsActions', 'serviceRequests', 'serviceVitalsSigns']; //es6 export for component export default VitalsCreateComponent;
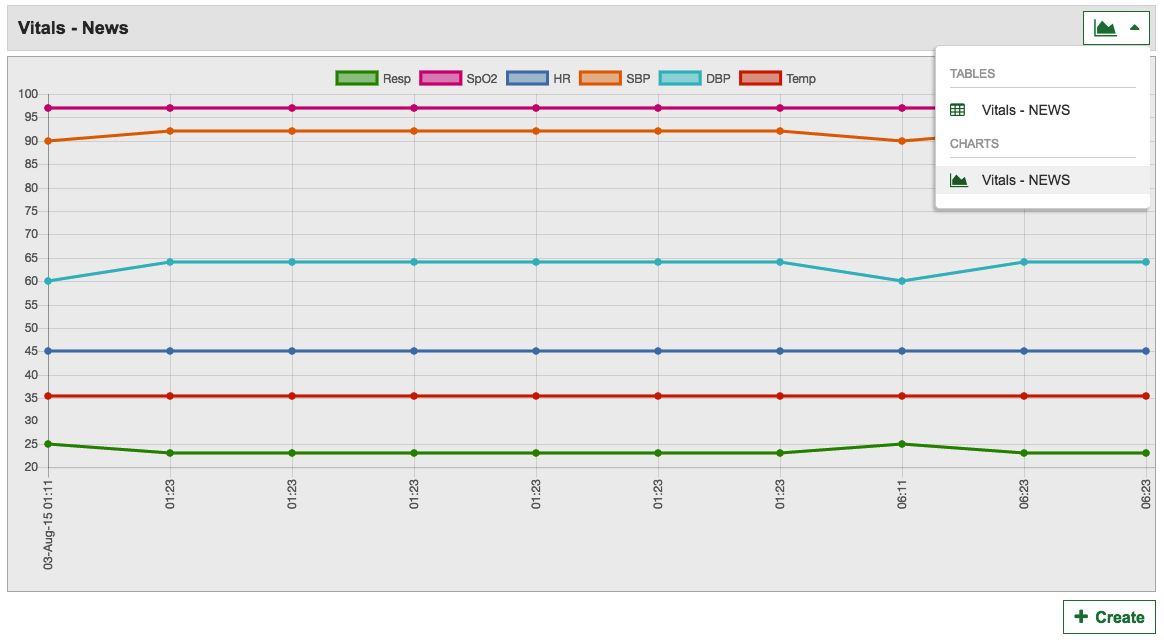
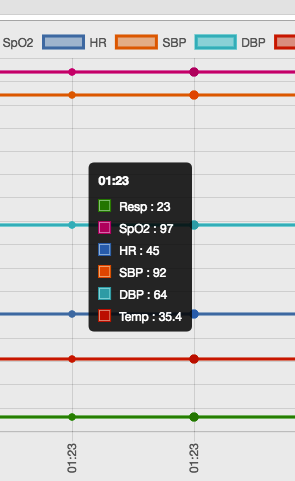
Vitals Actions
Component structure
//es6 import modules import {bindActionCreators} from 'redux'; import * as types from '../../../constants/ActionTypes'; //es6 export function export function all(patientId) { return { types: [types.VITALS, types.VITALS_SUCCESS, types.VITALS_ERROR], shouldCallAPI: (state) => !state.vitals.response, config: { method: 'get', url: '/api/patients/' + patientId + '/vitalsigns' }, meta: { timestamp: Date.now() } }; }
Vitals Reducer
Component structure
//es6 import modules import * as types from '../../../constants/ActionTypes'; const INITIAL_STATE = { isFetching: false, error: false, data: null, dataGet: null, dataCreate: null, dataUpdate: null }; //es6 export function export default function vitals(state = INITIAL_STATE, action) { const {payload} = action; //redux action for Vitals requests var actions = { [[types.VITALS]: (state) => { return Object.assign({}, state, { isFetching: true, error: false }); }