Referrals module
Referrals List
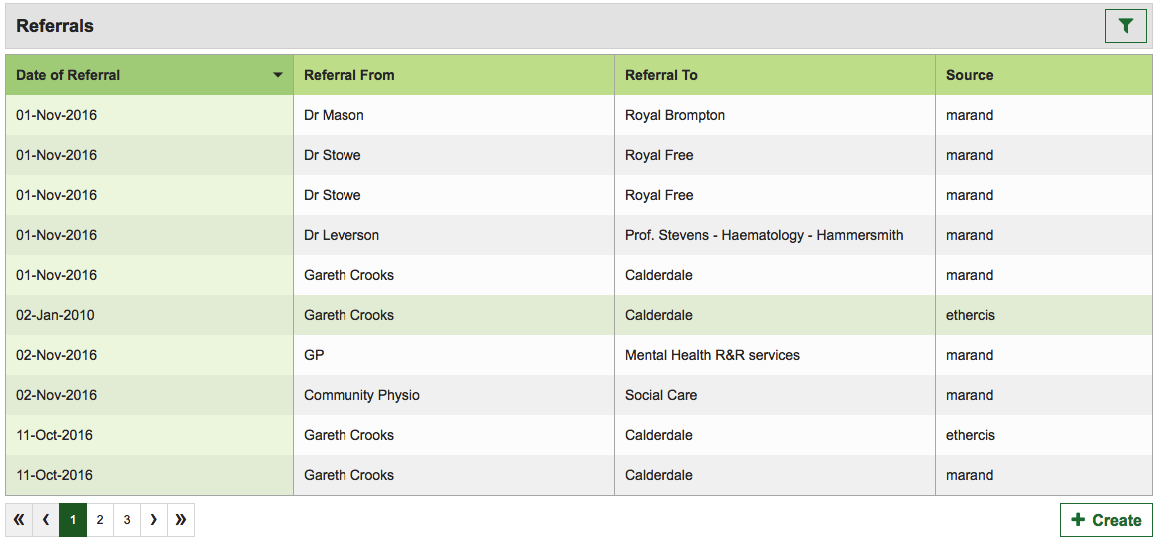
API URL
/api/patients/{patientId}/referrals
GET response
{ dateOfReferral:1458792662000 referralFrom:"Tony Shannon" referralTo:"Ripplefields Optometry service" source:"ethercis" sourceId:"94133578-f505-4e76-b4ed-762462508801" }
Component structure
//component template let templateReferralsList = require('./referrals-list.html'); //controller init class ReferralsListController { constructor($scope, $state, $stateParams, $ngRedux, referralsActions, serviceRequests, usSpinnerService, serviceFormatted) { } //component init const ReferralsListComponent = { template: templateReferralsList, controller: ReferralsListController }; //inject services/modules to controller ReferralsListController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'referralsActions', 'serviceRequests', 'usSpinnerService', 'serviceFormatted']; //es6 export for component export default ReferralsListComponent;
Referrals Detail
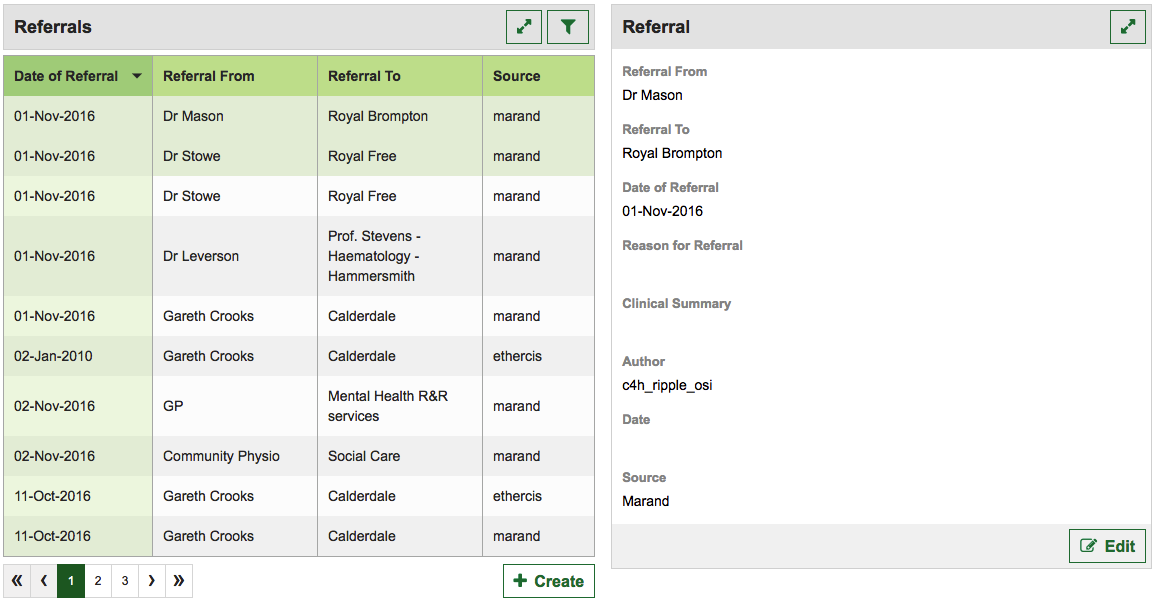
API URL
/api/patients/{patientId}/referrals/{sourceId}
GET response
{ author:"c4h_ripple_osi" dateOfReferral:1477994812035 referralCareFlow:"Service request sent" referralFrom:"Dr Mason" referralOutcome:"" referralRef:"816d8873-ccae-4e2c-bf42-4aee7ffcb802" referralServiceName:"Refer to Thoracic Surgeon" referralState:"planned" referralStateCode:526 referralStateDate:1477526400000 referralTo:"Royal Brompton" referralType:"Refer to Thoracic Surgeon" source:"Marand" sourceId:"aef53554-8e59-4c26-b4c3-b82ae1d2ddc4" }
Component structure
//component template let templateReferralsDetail= require('./referrals-detail.html'); //controller init class ReferralsDetailController { constructor($scope, $state, $stateParams, $ngRedux, referralsActions, usSpinnerService, serviceRequests) { } //component init const ReferralsDetailComponent = { template: templateReferralsDetail, controller: ReferralsDetailController }; //inject services/modules to controller ReferralsDetailController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'referralsActions', 'usSpinnerService', 'serviceRequests']; //es6 export for component export default ReferralsDetailComponent;
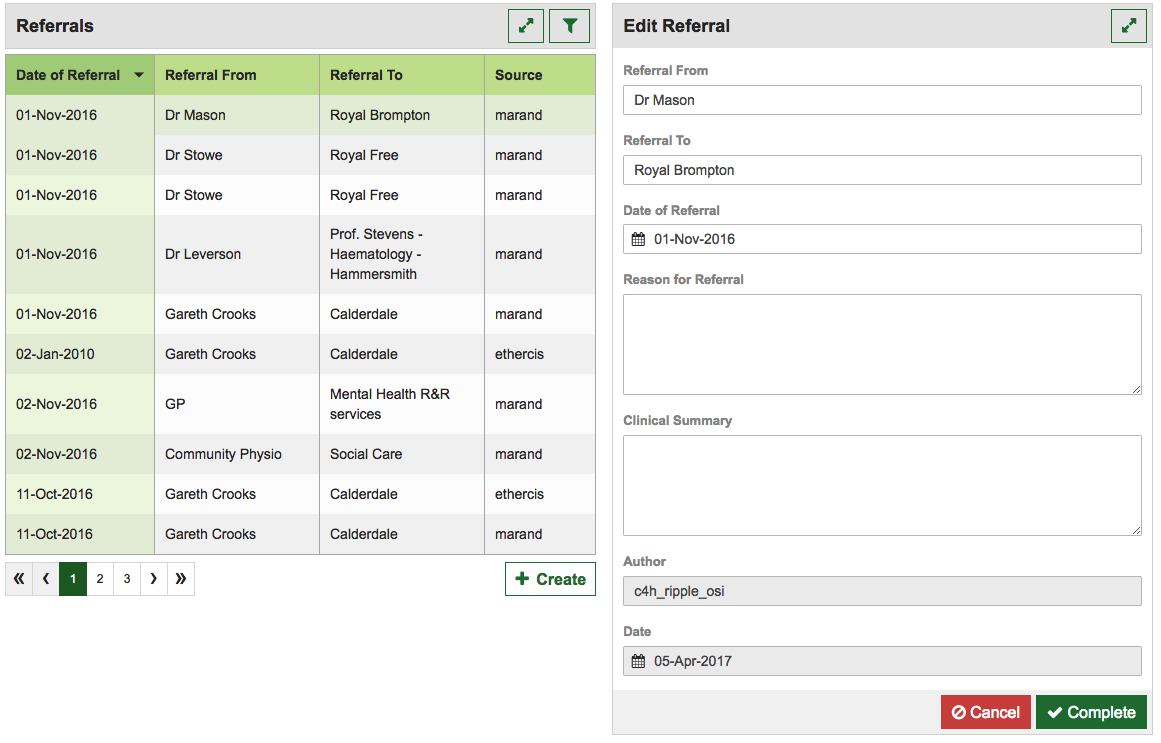
API URL
/api/patients/{patientId}/referrals
PUT data
{ author:"c4h_ripple_osi" clinicalSummary:"xxccv" dateCreated:"2017-04-07T13:06:44.937Z" dateOfReferral:1477994812035 reason:"zzzxxx" referralCareFlow:"Service request sent" referralFrom:"Dr Mason" referralOutcome:"" referralRef:"816d8873-ccae-4e2c-bf42-4aee7ffcb802" referralServiceName:"Refer to Thoracic Surgeon" referralState:"planned" referralStateCode:526 referralStateDate:1477526400000 referralTo:"Royal Brompton" referralType:"Refer to Thoracic Surgeon" source:"Marand" sourceId:"aef53554-8e59-4c26-b4c3-b82ae1d2ddc4" }
Referrals Create
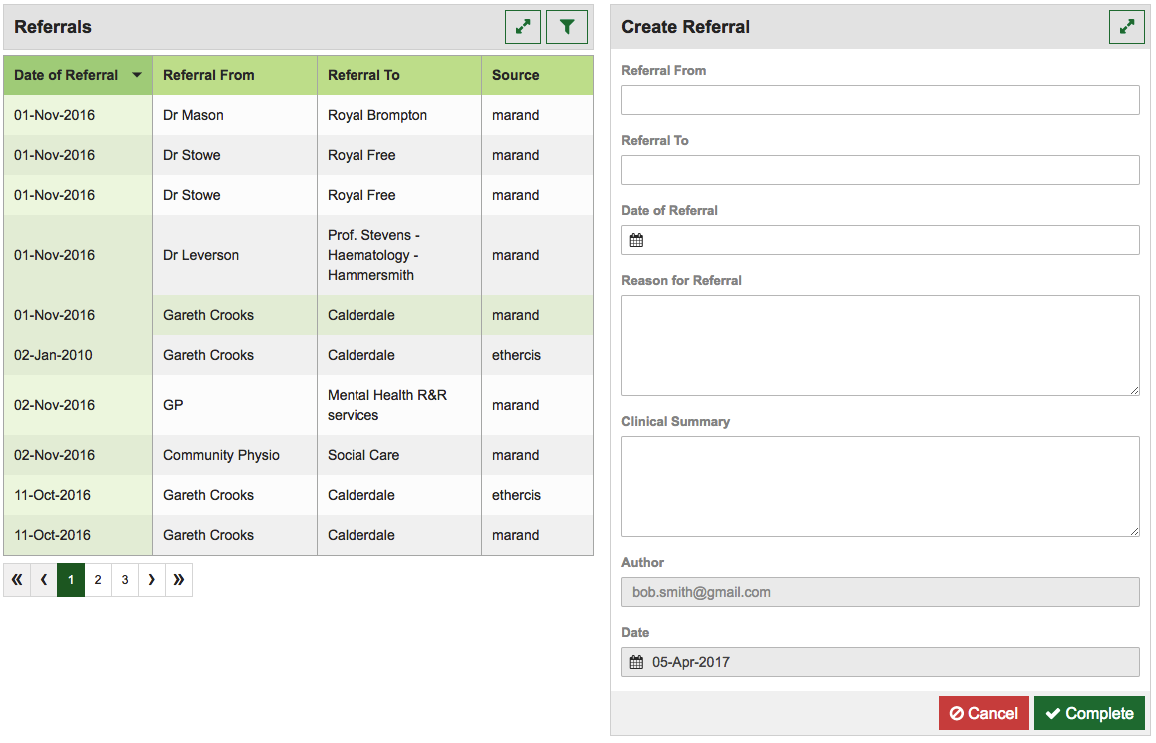
API URL
/api/patients/{patientId}/referrals
POST data
{ author:"c4h_ripple_osi" clinicalSummary:"xxccv" dateCreated:"2017-04-07T13:06:44.937Z" dateOfReferral:1477994812035 reason:"zzzxxx" referralCareFlow:"Service request sent" referralFrom:"Dr Mason" referralOutcome:"" referralRef:"816d8873-ccae-4e2c-bf42-4aee7ffcb802" referralServiceName:"Refer to Thoracic Surgeon" referralState:"planned" referralStateCode:526 referralStateDate:1477526400000 referralTo:"Royal Brompton" referralType:"Refer to Thoracic Surgeon" source:"Marand" }
Component structure
//component template let templateCreate= require('./referrals-create.html'); //controller init class ReferralsCreateController { constructor($scope, $state, $stateParams, $ngRedux, referralsActions, usSpinnerService, serviceRequests) { } //component init const ReferralsCreateComponent = { template: templateCreate, controller: ReferralsCreateController }; //inject services/modules to controller ReferralsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'referralsActions', 'usSpinnerService', 'serviceRequests']; //es6 export for component export default ReferralsCreateComponent;
Referrals Actions
Component structure
//es6 import modules import {bindActionCreators} from 'redux'; import * as types from '../../../constants/ActionTypes'; //es6 export function export function all(patientId) { return { types: [types.REFERRALS, types.REFERRALS_SUCCESS, types.REFERRALS_ERROR], shouldCallAPI: (state) => !state.referrals.response, config: { method: 'get', url: '/api/patients/' + patientId + '/referrals' }, meta: { timestamp: Date.now() } }; }
Referrals Reducer
Component structure
//es6 import modules import * as types from '../../../constants/ActionTypes'; const INITIAL_STATE = { isFetching: false, error: false, data: null, dataGet: null, dataCreate: null, dataUpdate: null }; //es6 export function export default function referrals(state = INITIAL_STATE, action) { const {payload} = action; //redux action for Referrals requests var actions = { [[types.REFERRALS]: (state) => { return Object.assign({}, state, { isFetching: true, error: false }); }