Clinical Notes module
Clinical Notes List
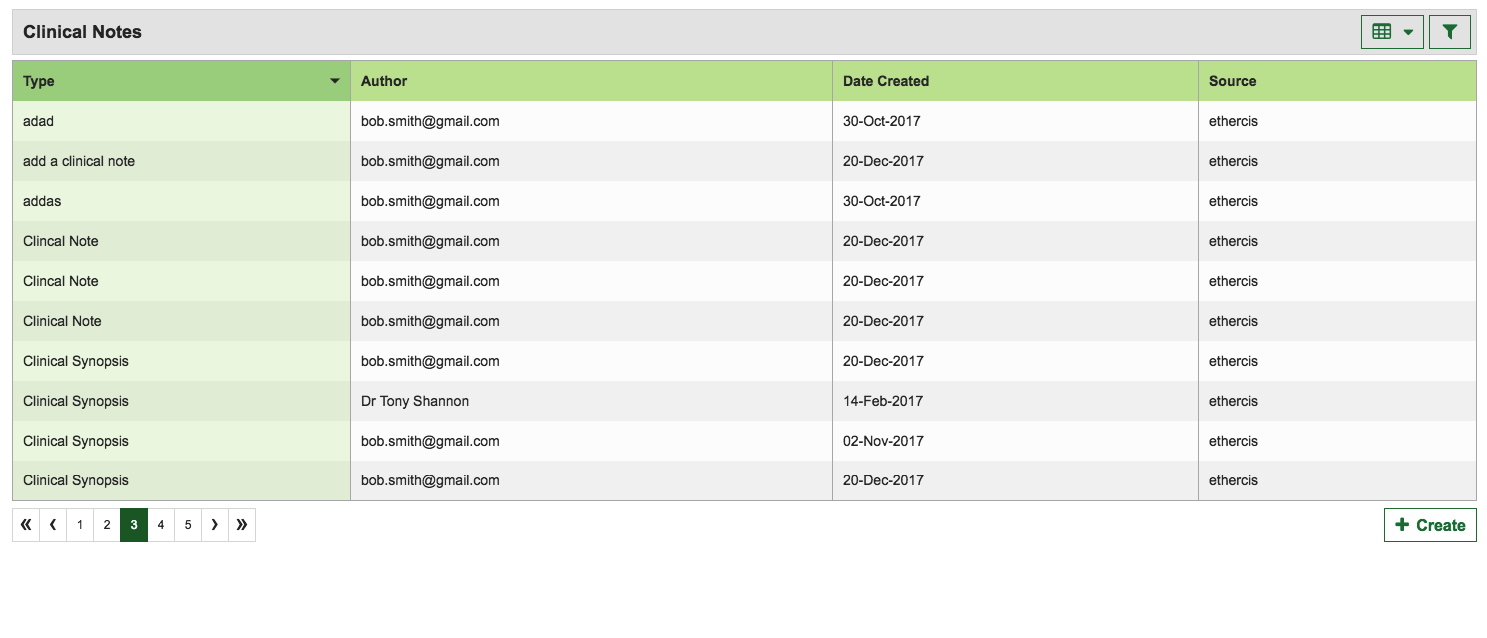
API URL
/api/patients/{patientId}/clinicalnotes
GET response
{ author:"Dr Tony Shannon" clinicalNotesType:"Clinical Synopsis" dateCreated:1487072396000 source:"ethercis" sourceId:"699160c0-e80e-466a-900c-2184a937bb57" }
Component structure
//component template let templateMedicationsCreate = require('./medications-create.html'); //controller init class MedicationsCreateController { constructor($scope, $state, $stateParams, $ngRedux, medicationsActions, serviceRequests, usSpinnerService) { } //component init const MedicationsCreateComponent = { template: templateMedicationsCreate, controller: MedicationsCreateController }; //inject services/modules to controller MedicationsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'medicationsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default MedicationsCreateComponent;
Clinical Notes Detail
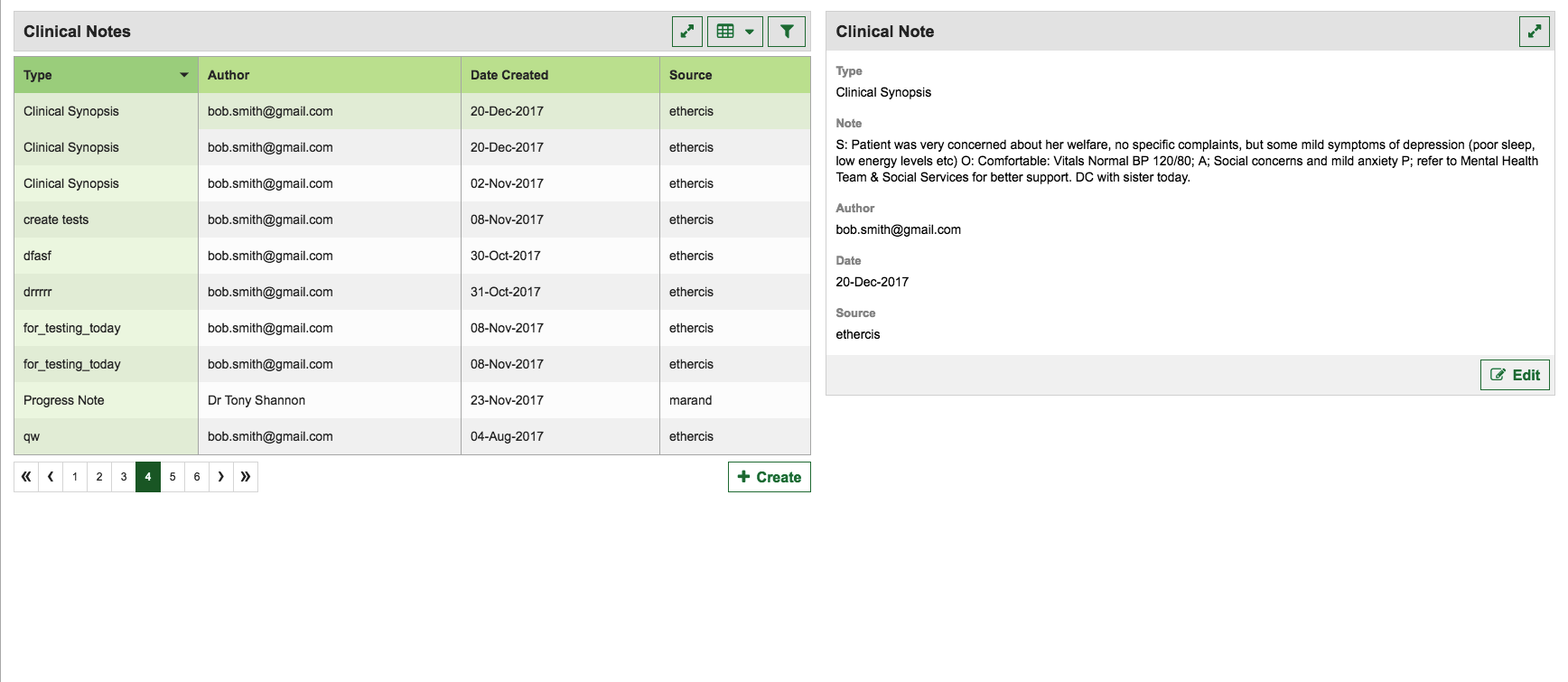
API URL
/api/patients/{patientId}/clinicalnotes/{sourceId}
GET response
{ author:"Dr Tony Shannon" clinicalNotesType:"SOAP Note" dateCreated:1456287062000 note:"S: Patient was very concerned about her welfare" source:"EtherCIS" sourceId:"3e93aeb5-1d5d-4e5c-b249-4ce34eec6d84" }
Component structure
//component template let templateMedicationsCreate = require('./medications-create.html'); //controller init class MedicationsCreateController { constructor($scope, $state, $stateParams, $ngRedux, medicationsActions, serviceRequests, usSpinnerService) { } //component init const MedicationsCreateComponent = { template: templateMedicationsCreate, controller: MedicationsCreateController }; //inject services/modules to controller MedicationsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'medicationsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default MedicationsCreateComponent;
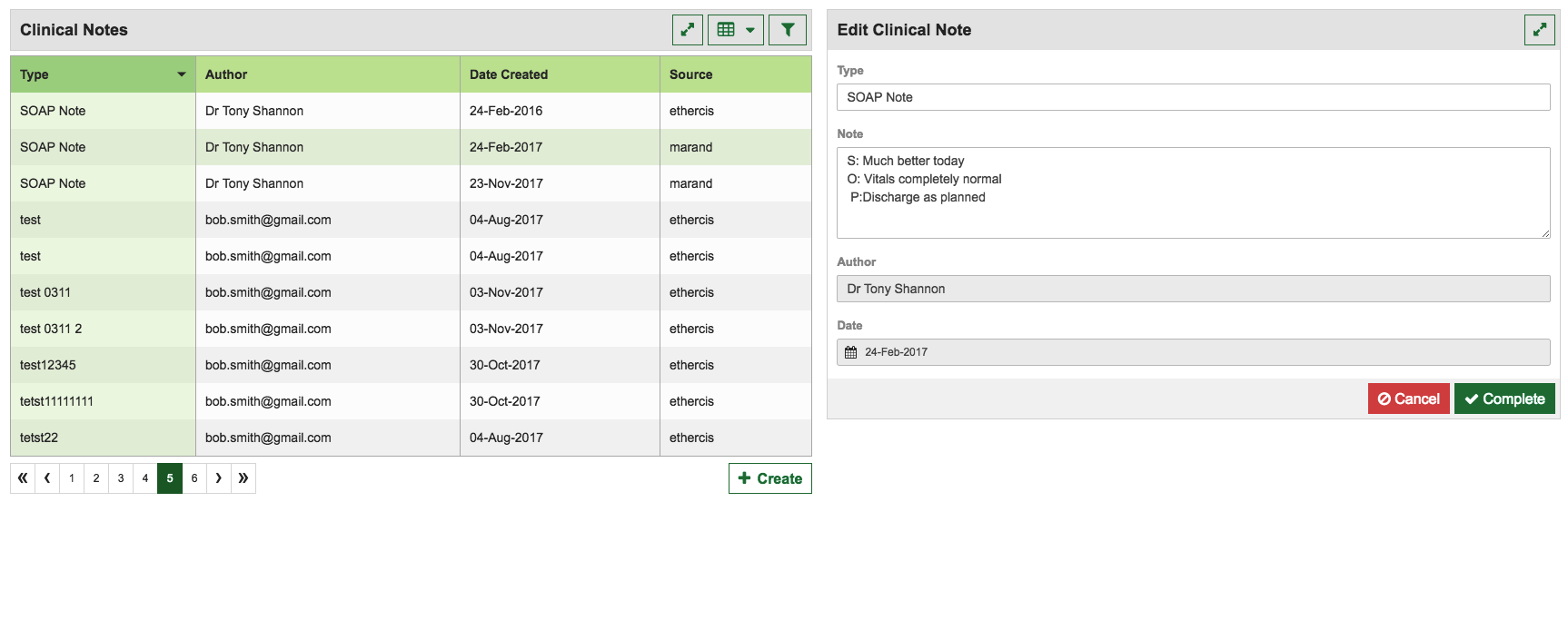
API URL
/api/patients/{patientId}/clinicalnotes
PUT data
{ author:"Dr Tony Shannon" noteType:"SOAP Note t" notes:"S: Patient was very concerned about her welfare" source:"EtherCIS" sourceId:"3e93aeb5-1d5d-4e5c-b249-4ce34eec6d84" }
Clinical Notes Create
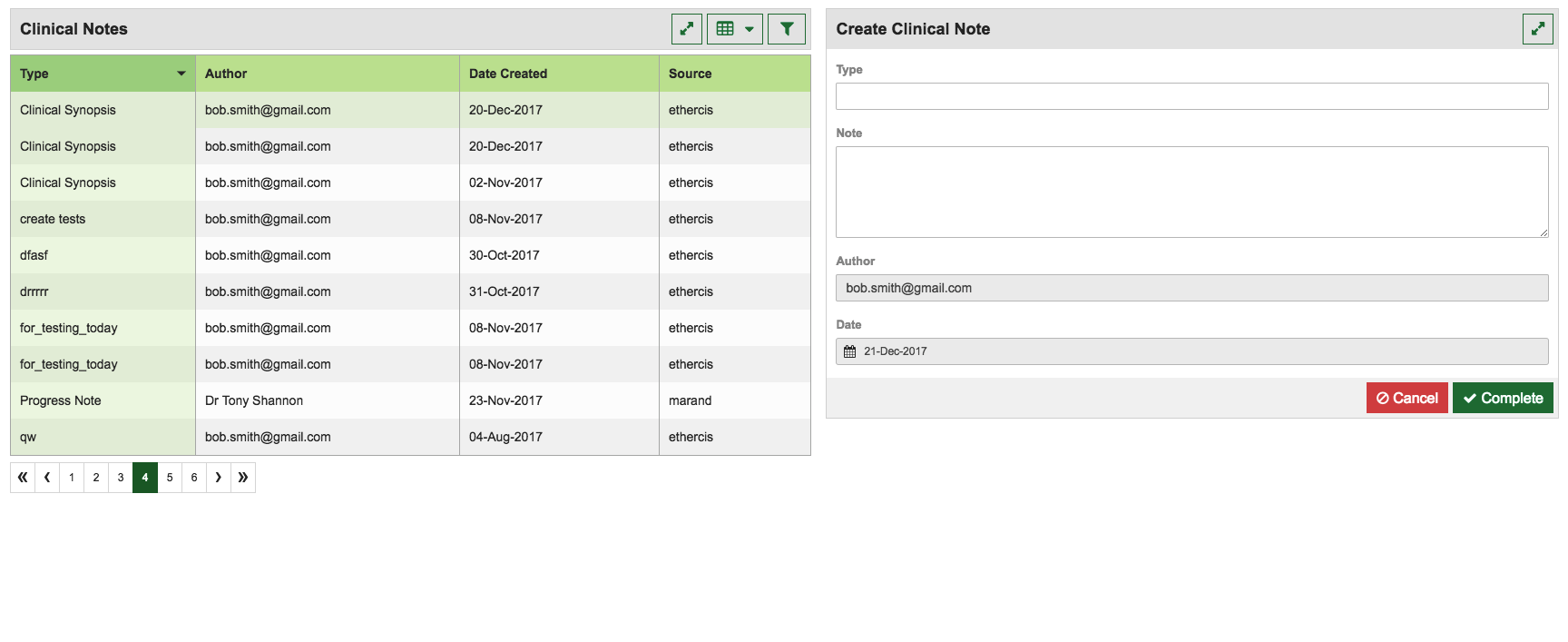
API URL
/api/patients/{patientId}/clinicalnotes
POST data
{ dateCreated:"2017-04-07" noteType:"test note" notes:"qwerty" source:"openehr" }
Component structure
//component template let templateMedicationsCreate = require('./medications-create.html'); //controller init class MedicationsCreateController { constructor($scope, $state, $stateParams, $ngRedux, medicationsActions, serviceRequests, usSpinnerService) { } //component init const MedicationsCreateComponent = { template: templateMedicationsCreate, controller: MedicationsCreateController }; //inject services/modules to controller MedicationsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'medicationsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default MedicationsCreateComponent;
Clinical Notes Actions
Component structure
//es6 import modules import {bindActionCreators} from 'redux'; import * as types from '../../../constants/ActionTypes'; //es6 export function export function all(patientId) { return { types: [types.CLINICALNOTES, types.CLINICALNOTES_SUCCESS, types.CLINICALNOTES_ERROR], shouldCallAPI: (state) => !state.clinicalnotes.response, config: { method: 'get', url: '/api/patients/' + patientId + '/clinicalnotes' }, meta: { timestamp: Date.now() } }; }
Clinical Notes Reducer
Component structure
//es6 import modules import * as types from '../../../constants/ActionTypes'; const INITIAL_STATE = { isFetching: false, error: false, data: null, dataGet: null, dataCreate: null, dataUpdate: null }; //es6 export function export default function clinicalnotes(state = INITIAL_STATE, action) { const {payload} = action; //redux action for Clinical notes requests var actions = { [types.CLINICALNOTES]: (state) => { state.dataCreate = null; state.dataUpdate = null; return Object.assign({}, state, { isFetching: true, error: false }); } }