Drawings module
Drawings List

API URL
/api/patients/{patientId}/drawings
GET response
{ date: 1423440000000 name: 'Cardiac Catherization' source: 'Marand' sourceId: "6ba725c8-9a61-41e9-82c0-d4c6788d9ec8" }
Component structure
//component template let templateDrawingsList = require('./drawings-list.html'); //controller init class DrawingsListController { constructor($scope, $state, $stateParams, $ngRedux, drawingsActions, serviceRequests, usSpinnerService, serviceFormatted) { } //component init const DrawingsListComponent = { template: templateDrawingsList, controller: DrawingsListController }; //inject services/modules to controller DrawingsListController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'drawingsActions', 'serviceRequests', 'usSpinnerService', 'serviceFormatted']; //es6 export for component export default DrawingsListComponent;
Drawings Detail
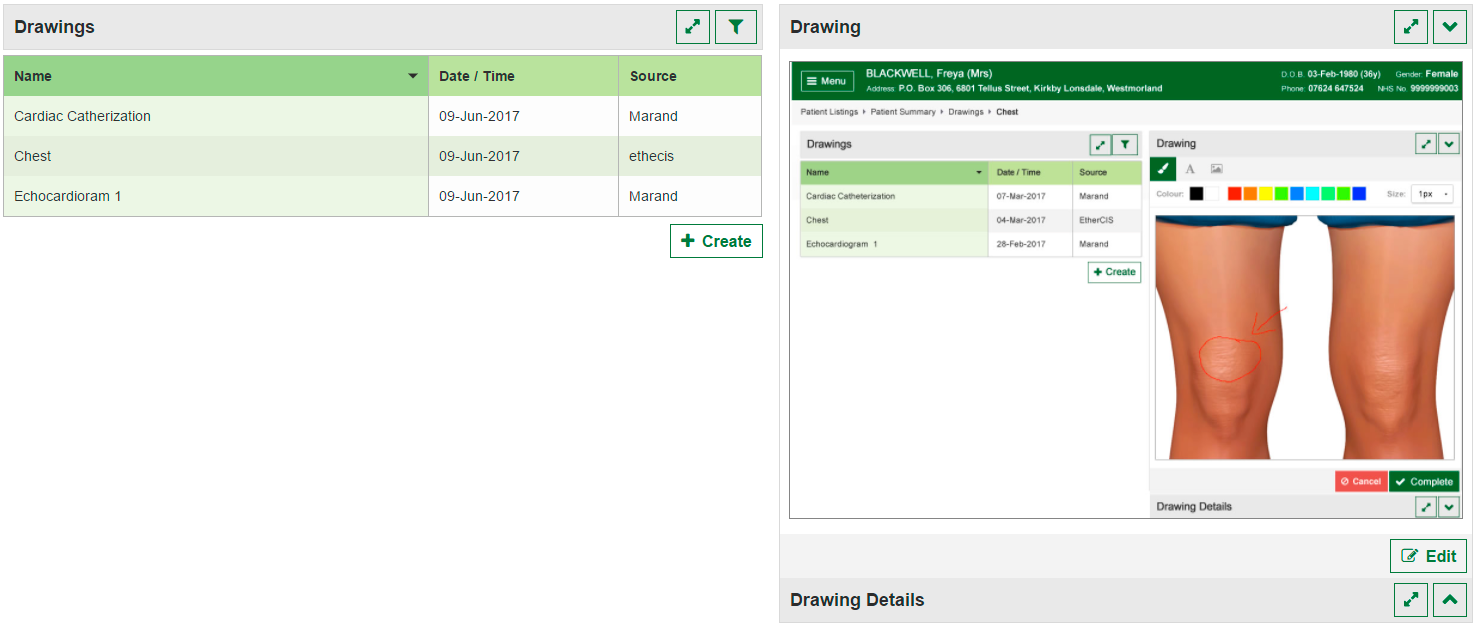
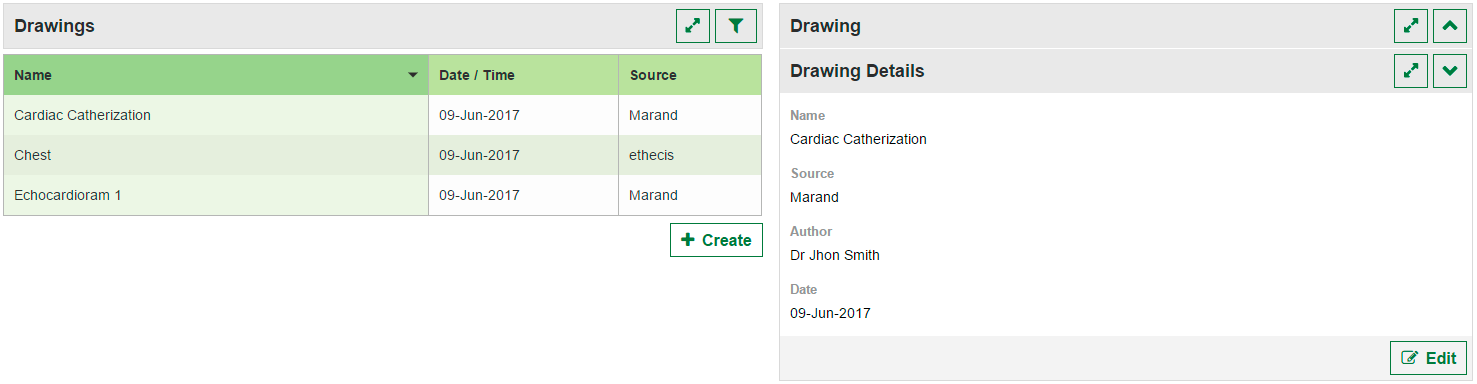
API URL
/api/patients/{patientId}/drawings/{sourceId}
GET response
{ author: 'Dr Jhon Smith' date: 1423440000000 image64: 'data:image/png;base64,/...' name: 'Cardiac Catherization' source: 'Marand' sourceId: "6ba725c8-9a61-41e9-82c0-d4c6788d9ec8" }
Component structure
//component template let templateDrawingsDetail= require('./drawings-detail.html'); //controller init class DrawingsDetailController { constructor($scope, $state, $stateParams, $ngRedux, drawingsActions, usSpinnerService, serviceRequests, $timeout, $window) { } //component init const DrawingsDetailComponent = { template: templateDrawingsDetail, controller: DrawingsDetailController }; //inject services/modules to controller DrawingsDetailController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'drawingsActions', 'usSpinnerService', 'serviceRequests', '$timeout', '$window']; //es6 export for component export default DrawingsDetailComponent;
Drawings Create
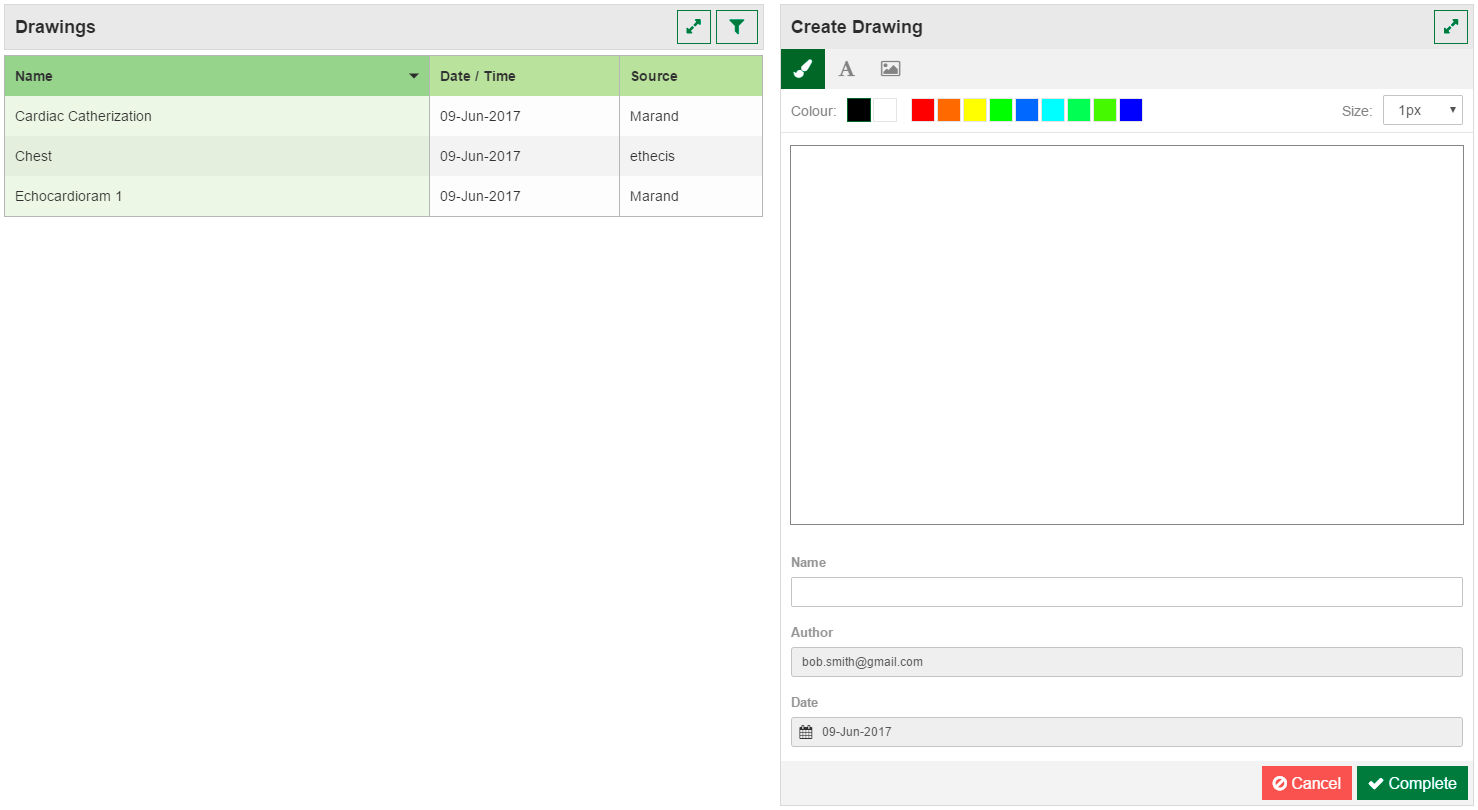
API URL
/api/patients/{patientId}/drawings
POST data
{ author: 'Dr Jhon Smith' date: 1423440000000 image64: 'data:image/png;base64,/...' name: 'Cardiac Catherization' }
Component structure
//component template let templateCreate = require('./drawings-create.html'); //controller init class DrawingsCreateController { constructor($scope, $state, $stateParams, $ngRedux, drawingsActions, serviceRequests, serviceFormatted, usSpinnerService, $window) { } //component init const DrawingsCreateComponent = { template: templateCreate, controller: DrawingsCreateController }; //inject services/modules to controller DrawingsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'drawingsActions', 'serviceRequests', 'serviceFormatted', 'usSpinnerService', '$window']; //es6 export for component export default DrawingsCreateComponent;
Drawings Create
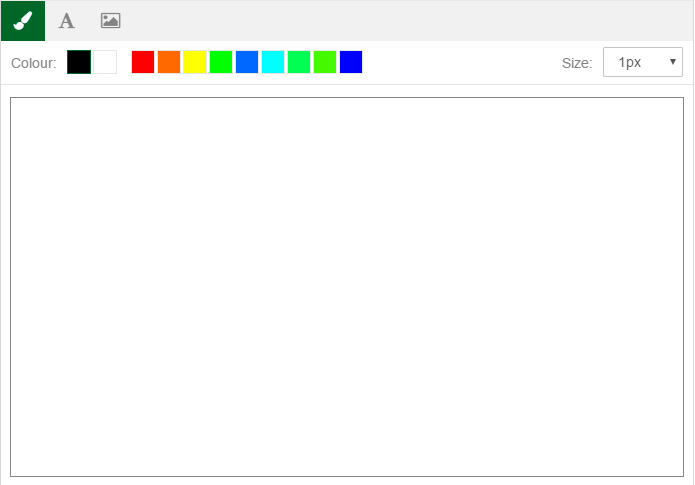
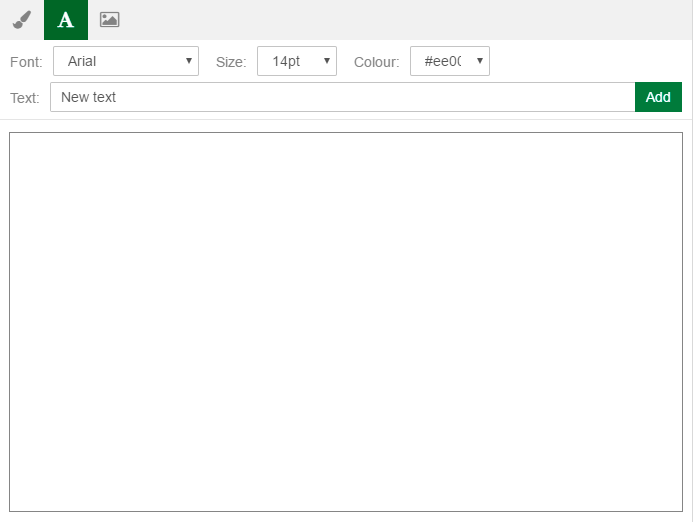
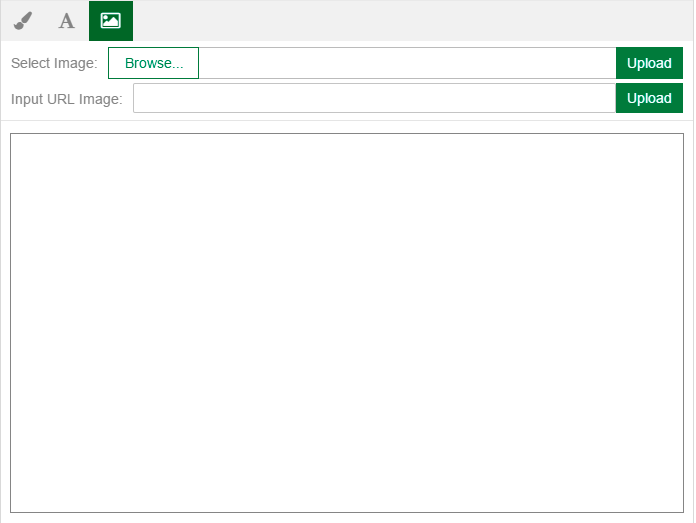
Component structure
//component template let templateDrawingsDrawing = require('./drawings-drawing.html'); //controller init class DrawingsDrawingController { constructor($scope, $state, $stateParams, serviceRequests, usSpinnerService, $timeout, $window) { } //component init const DrawingsDrawingComponent = { template: templateDrawingsDrawing, controller: DrawingsDrawingController }; //inject services/modules to controller DrawingsDrawingController.$inject = ['$scope', '$state', '$stateParams', 'serviceRequests', 'usSpinnerService', '$timeout', '$window']; //es6 export for component export default DrawingsDrawingComponent;
Drawings Actions
Component structure
//es6 import modules import {bindActionCreators} from 'redux'; import * as types from '../../../constants/ActionTypes'; //es6 export function export function all(patientId) { return { types: [types.DRAWINGS, types.DRAWINGS_SUCCESS, types.DRAWINGS_ERROR], shouldCallAPI: (state) => !state.drawings.response, config: { method: 'get', url: '/api/patients/' + patientId + '/drawings' }, d: { timestamp: Date.now() } }; }
Drawings Reducer
Component structure
//es6 import modules import * as types from '../../../constants/ActionTypes'; const INITIAL_STATE = { isFetching: false, error: false, data: null, dataGet: null, dataCreate: null }; //es6 export function export default function drawing(state = INITIAL_STATE, action) { const {payload} = action; //redux action for Drawings requests [types.DRAWINGS]: (state) => { state.dataCreate = null; state.dataUpdate = null; return Object.assign({}, state, { isFetching: true, error: false }); }