Contacts module
Contacts List
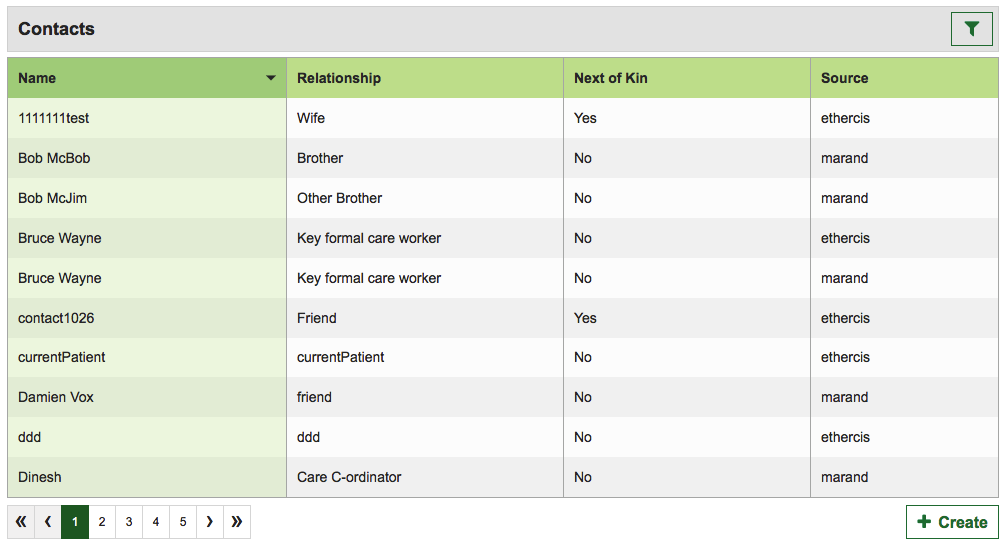
API URL
/api/patients/{patientId}/contacts
GET response
{ name:"Name" nextOfKin:true relationship:"Name" source:"ethercis" sourceId:"8f8d258c-9c6d-4b94-94f9-c38c58363bbb" }
Component structure
//component template let templateContactsList = require('./contacts-list.html'); //controller init class ContactsListController { constructor($scope, $state, $stateParams, $ngRedux, contactsActions, serviceRequests, usSpinnerService) { } //component init const ContactsListComponent = { template: templateContactsList, controller: ContactsListController }; //inject services/modules to controller ContactsListController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'contactsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default ContactsListComponent;
Contacts Detail
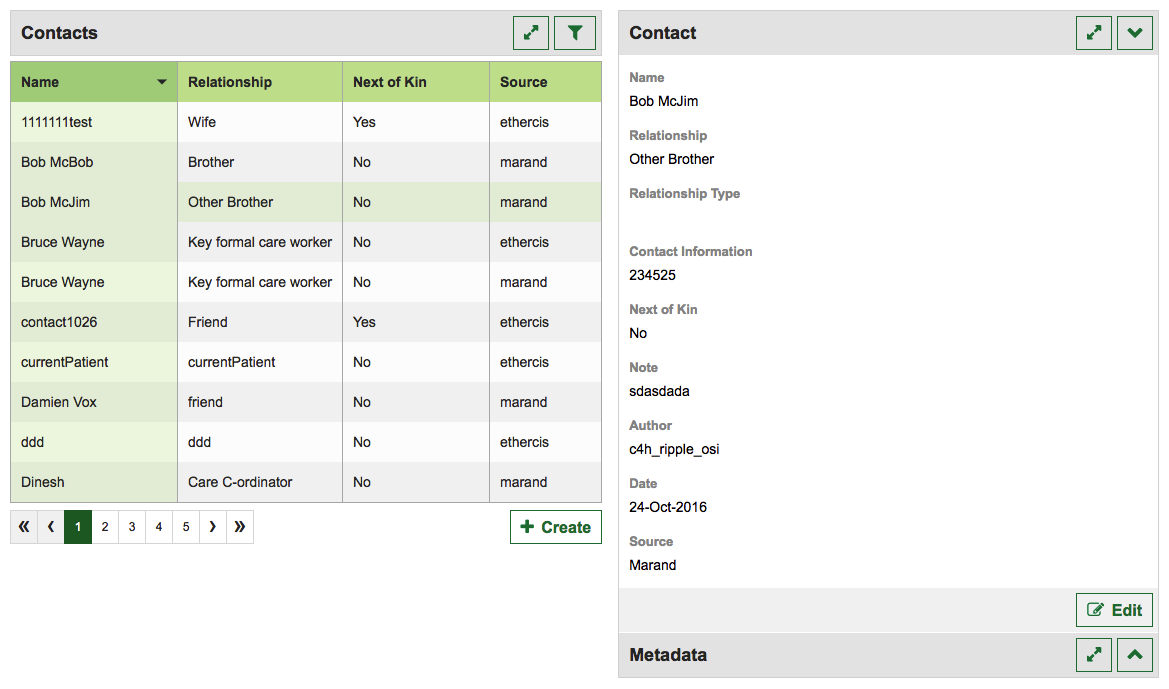
API URL
/api/patients/{patientId}/contacts/{sourceId}
GET response
{ author:"c4h_ripple_osi" contactInformation:234525 dateCreated:1477267200000 name:"Bob McJim" nextOfKin:"" notes:"sdasdada" relationship:"Other Brother" relationshipCode:"" relationshipTerminology:"" relationshipType:"" source:"Marand" sourceId:"7b6bf9f1-d05b-4d4e-85e3-c120506bb7d3" }
Component structure
//component template let templateContactsDetail= require('./contacts-detail.html'); //controller init class ContactsDetailController { constructor($scope, $state, $stateParams, $ngRedux, patientsActions, contactsActions, serviceRequests, usSpinnerService) { } //component init const ContactsDetailComponent = { template: templateContactsDetail, controller: ContactsDetailController }; //inject services/modules to controller ContactsDetailController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'patientsActions', 'contactsActions', 'serviceRequests', 'usSpinnerService']; //es6 export for component export default ContactsDetailComponent;
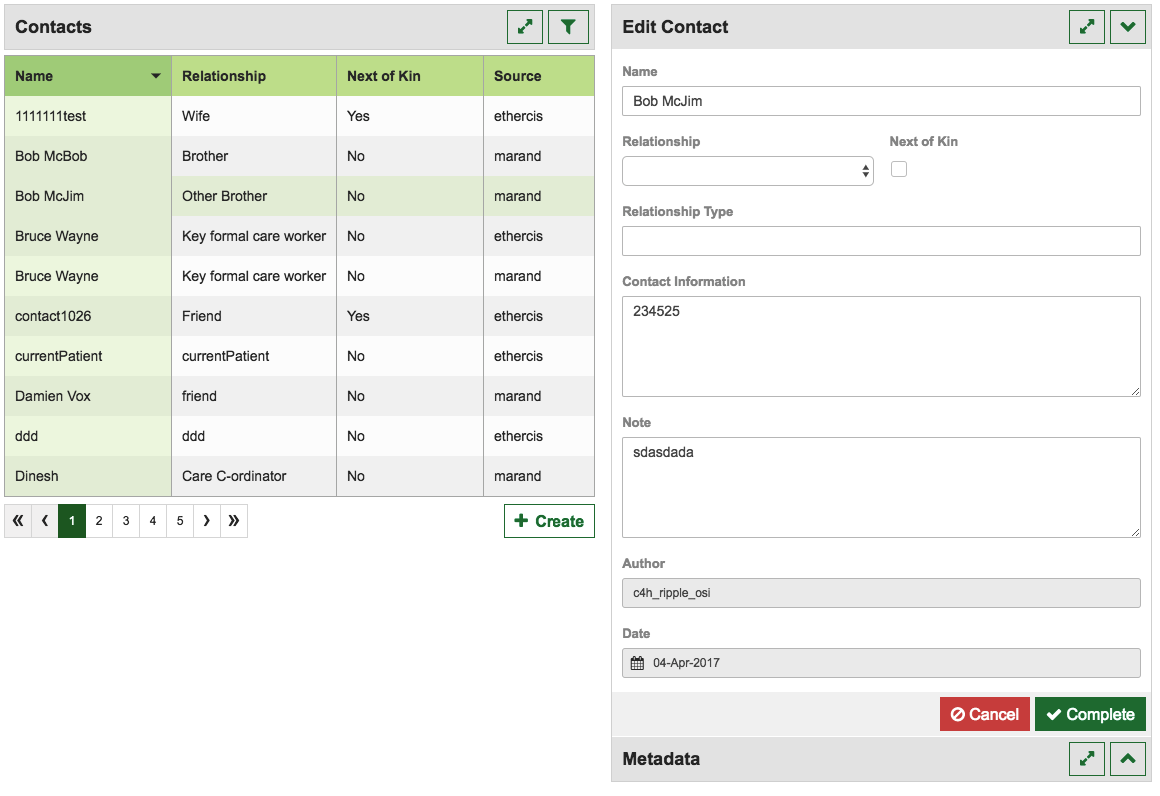
API URL
/api/patients/{patientId}/contacts/{sourceId}
PUT data
{ author:"c4h_ripple_osi" contactInformation:234525 dateCreated:1477267200000 dateSubmitted:"2017-04-06T13:59:56.672Z" name:"Bob McJim" nextOfKin:"" notes:"sdasdada" relationship:"Father" relationshipCode:"" relationshipTerminology:"" relationshipType:"qqq" source:"Marand" sourceId:"7b6bf9f1-d05b-4d4e-85e3-c120506bb7d3" }
Contacts Create
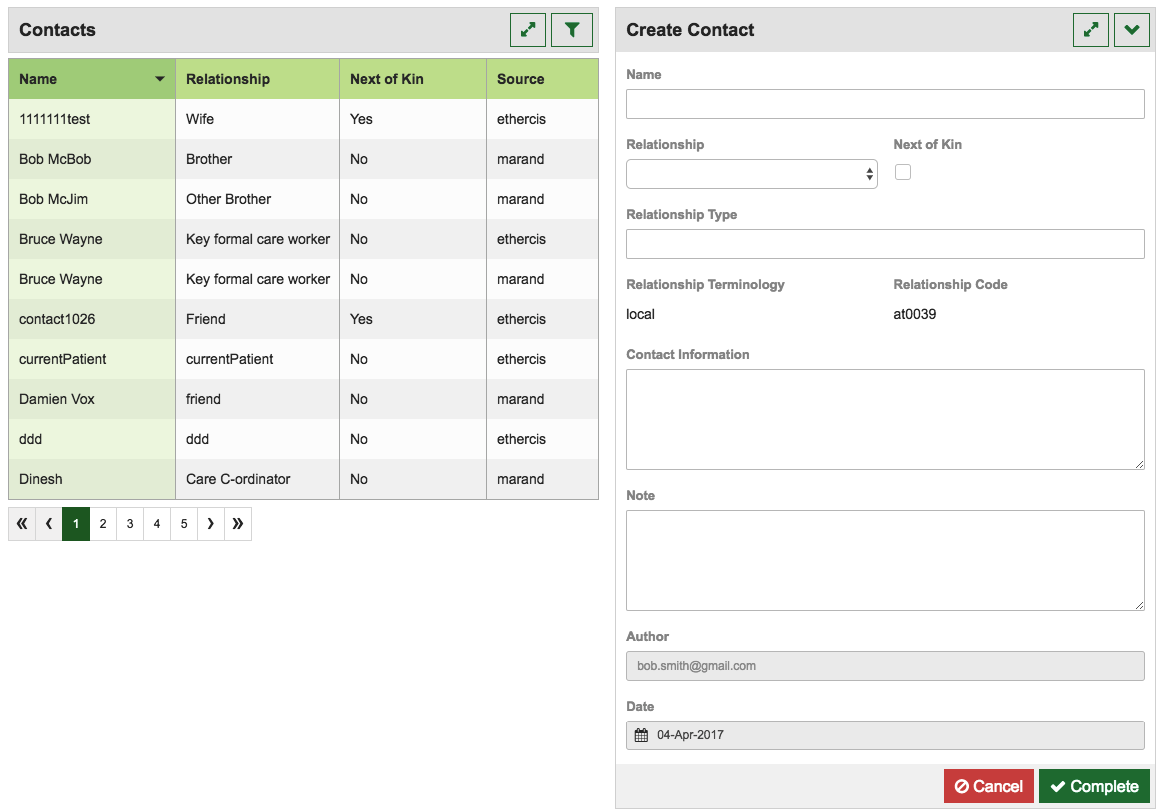
API URL
/api/patients/{patientId}/contacts/{sourceId}
POST data
{ contactInformation:"qqq" dateSubmitted:"2017-04-06T14:01:09.917Z" name:"test name" notes:"qqq" relationship:"Father" relationshipCode:"at0039" relationshipTerminology:"local" relationshipType:"qqq" }
Component structure
//component template let templateContactsCreate= require('./contacts-create.html'); //controller init class ContactsCreateController { constructor($scope, $state, $stateParams, $ngRedux, patientsActions, contactsActions, serviceRequests) { } //component init const ContactsCreateComponent = { template: templateContactsCreate, controller: ContactsCreateController }; //inject services/modules to controller ContactsCreateController.$inject = ['$scope', '$state', '$stateParams', '$ngRedux', 'patientsActions', 'contactsActions', 'serviceRequests']; //es6 export for component export default ContactsCreateComponent;