Clinical Statements module
Clinical Statements
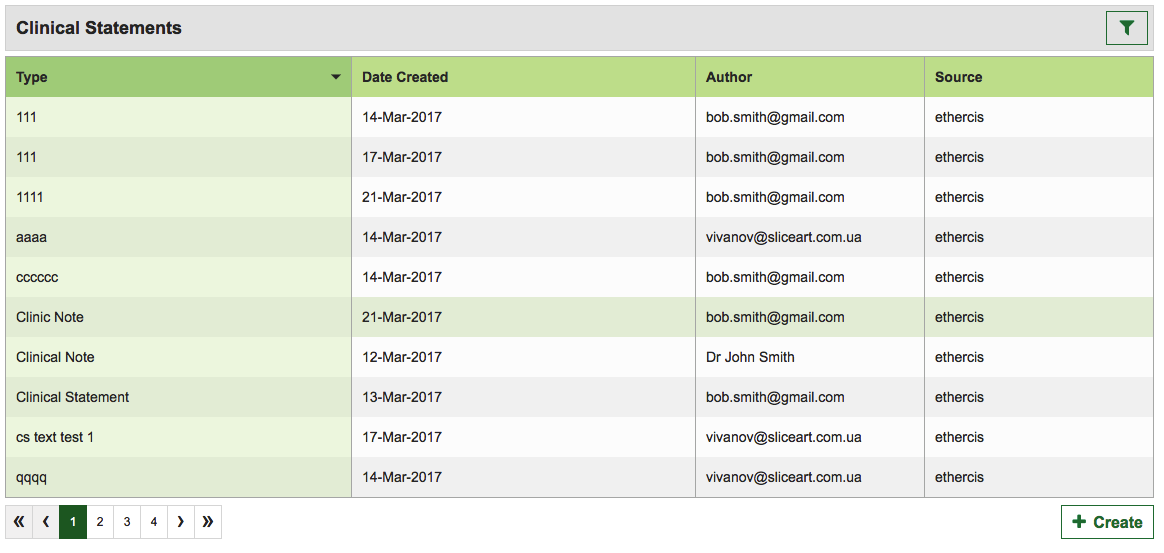
API URL
/api/patients/{patientId}/clinicalStatements
GET response
{ author:"bob.smith@gmail.com" dateCreated:1489655645844 source:"ethercis" sourceId:"0f86daea-9d25-4921-aa9c-b79b6478433d" type:"test ids" }
Component structure
// import packages import React, { PureComponent } from 'react'; import { bindActionCreators } from 'redux'; import { connect } from 'react-redux'; import { lifecycle, compose } from 'recompose'; import PluginListHeader from '../../plugin-page-component/PluginListHeader'; import PluginMainPanel from '../../plugin-page-component/PluginMainPanel'; import { fetchPatientClinicalStatementsRequest } from './ducks/fetch-patient-clinical-statements.duck'; import { fetchPatientClinicalStatementsCreateRequest } from './ducks/fetch-patient-clinical-statements-create.duck'; import { fetchPatientClinicalStatementsDetailRequest } from './ducks/fetch-patient-clinical-statements-detail.duck'; import { fetchPatientClinicalStatementsOnMount, fetchPatientClinicalStatementsDetailOnMount } from '../../../utils/HOCs/fetch-patients.utils'; import { patientClinicalStatementsSelector, clinicalStatementsCreateFormStateSelector, patientClinicalStatementsDetailSelector } from './selectors'; import ClinicalStatementsDetail from './ClinicalStatementsDetail/ClinicalStatementsDetail'; import PluginCreate from '../../plugin-page-component/PluginCreate'; import ClinicalStatementsCreateForm from './ClinicalStatementsCreate/ClinicalStatementsCreateForm' // map dispatch to Properties const mapDispatchToProps = dispatch => ({ actions: bindActionCreators({ fetchPatientClinicalStatementsRequest, fetchPatientClinicalStatementsCreateRequest, fetchPatientClinicalStatementsDetailRequest }, dispatch) }); // Higher-Order Components (HOC) for get some data @connect(patientClinicalStatementsSelector, mapDispatchToProps) @connect(patientClinicalStatementsDetailSelector) @connect(clinicalStatementsCreateFormStateSelector) @compose(lifecycle(fetchPatientClinicalStatementsOnMount), lifecycle(fetchPatientClinicalStatementsDetailOnMount)) export default class ClinicalStatements extends PureComponent { // React component // component template render() { return () } }
Clinical Statements Detail
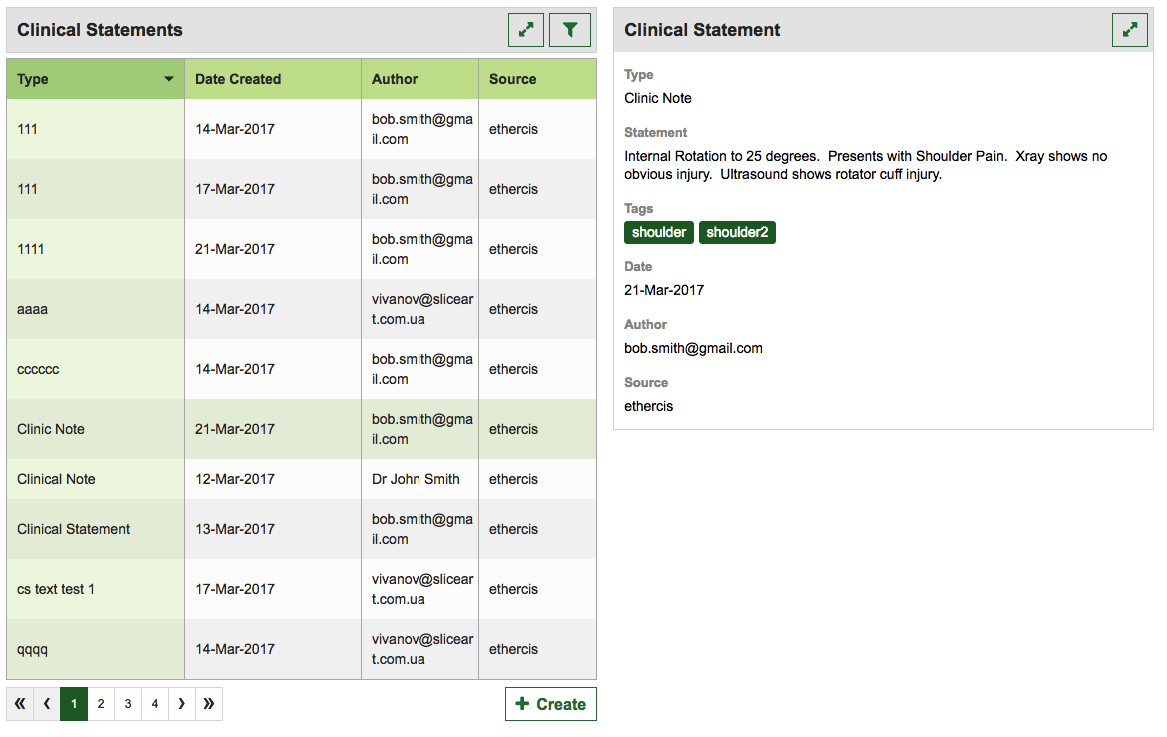
API URL
/api/patients/{patientId}/clinicalStatements/{sourceId}
GET response
{ author:"bob.smith@gmail.com" dateCreated:1489489481713 source:"ethercis" sourceId:"f546b8c0-97d6-489f-97e1-02622d721dc2" text:"The pain was medium at 5/10 in severity. " type:"aaaa" }
Component structure
// import packages import React, { PureComponent } from 'react'; import PluginDetailPanel from '../../../plugin-page-component/PluginDetailPanel'; import { Tag, TagList } from '../../../ui-elements/Tag/Tag'; import { getDDMMMYYYY } from '../../../../utils/time-helpers.utils'; import { valuesNames, valuesLabels } from '../forms.config'; export default class ClinicalStatementsDetail extends PureComponent { // React component // component template render() { return () } }
Clinical Statements Create Form
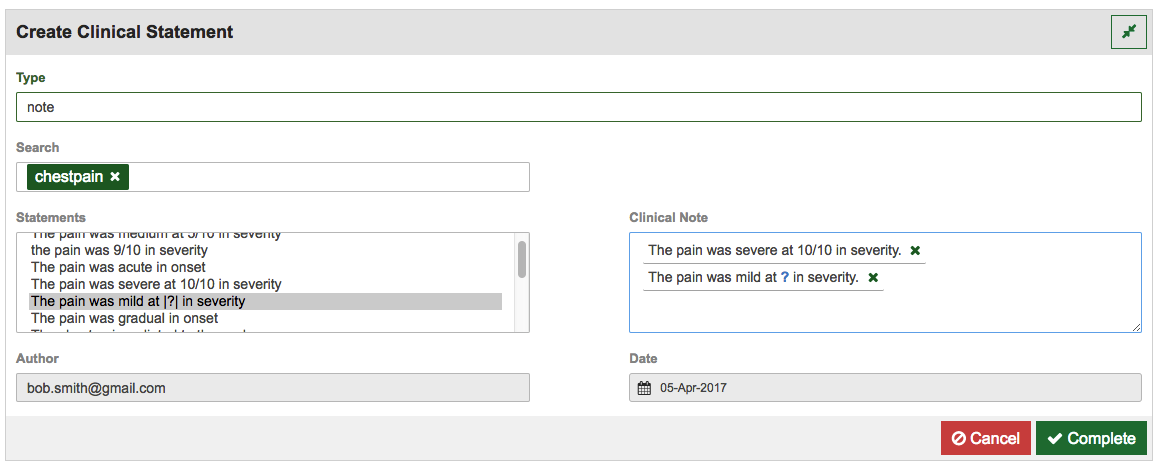
API URL
/api/patients/{patientId}/clinicalStatements
POST data
{
author:"bob.smith@gmail.com"
contentStore:{name: "ts", phrases: [{id: "6", tag: "chestpain"}]}
dateCreated:"2017-04-07T09:49:29.918Z"
text:'The pain was severe at 10/10 in severity. '
type:"test CS 33"
}
Component structure
// import packages import React, { PureComponent } from 'react'; import { Field, reduxForm } from 'redux-form'; import ValidatedInput from '../../../form-fields/ValidatedInputFormGroup'; import ClinicalNoteField from '../form-fields/ClinicalNoteField'; import DateInput from '../../../form-fields/DateInput'; import { validateForm } from '../forms.validation'; import { valuesNames, valuesLabels } from '../forms.config'; import { defaultFormValues } from './default-values.config'; import { getDDMMMYYYY } from '../../../../utils/time-helpers.utils'; // decorator to connect its form component to Redux @reduxForm({ form: 'clinicalStatementsCreateFormSelector', validate: validateForm, }) export default class ClinicalStatementsCreateForm extends PureComponent { // React component // component template render() { return () } }
Clinical Statements Clinical Note Field
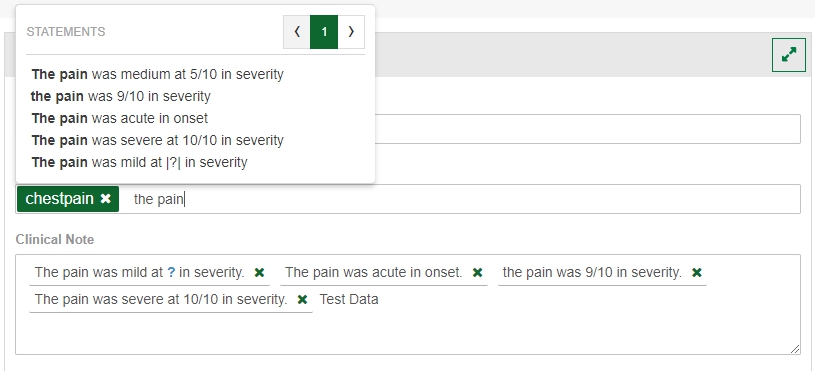
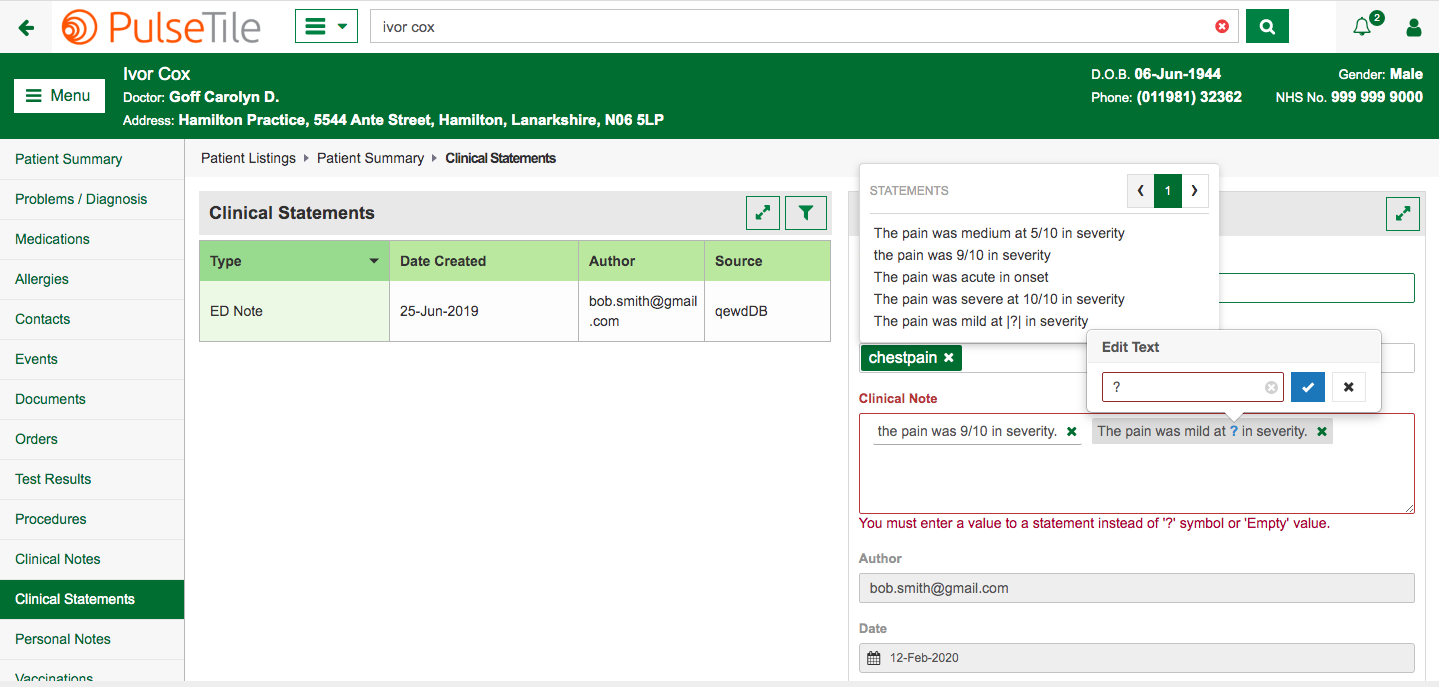
Component structure
// import packages import React, { PureComponent } from 'react'; import { bindActionCreators } from 'redux'; import { connect } from 'react-redux'; import { lifecycle, compose } from 'recompose'; import PropTypes from 'prop-types'; import classNames from 'classnames'; import 'bootstrap'; import 'x-editable/dist/bootstrap3-editable/js/bootstrap-editable'; import 'x-editable/dist/bootstrap3-editable/css/bootstrap-editable.css'; import PaginationBlock from '../../../presentational/PaginationBlock/PaginationBlock'; import { valuesLabels, valuesNames } from '../forms.config'; import { operationsOnCollection } from '../../../../utils/plugin-helpers.utils'; import * as helper from './clinical-statements-helper'; import { fetchPatientClinicalStatementsTagsRequest } from '../ducks/fetch-patient-clinical-statements-tags.duck'; import { fetchPatientClinicalStatementsQueryRequest } from '../ducks/fetch-patient-clinical-statements-query.duck'; import { fetchPatientClinicalStatementsTagsOnMount } from '../../../../utils/HOCs/fetch-patients.utils'; import { patientClinicalStatementsTagsSelector, patientClinicalStatementsQuerySelector } from '../selectors'; // map dispatch to Properties const mapDispatchToProps = dispatch => ({ actions: bindActionCreators({ fetchPatientClinicalStatementsTagsRequest, fetchPatientClinicalStatementsQueryRequest }, dispatch) }); // Higher-Order Components (HOC) for get some data @connect(patientClinicalStatementsTagsSelector, mapDispatchToProps) @connect(patientClinicalStatementsQuerySelector) @compose(lifecycle(fetchPatientClinicalStatementsTagsOnMount)) export default class ClinicalNoteField extends PureComponent { // React component // component template render() { return () } }
Clinical Statements List Duck
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; import { handleErrors } from '../../../../ducks/handle-errors.duck'; // Actions names export const FETCH_PATIENT_CLINICAL_STATEMENTS_REQUEST = 'FETCH_PATIENT_CLINICAL_STATEMENTS_REQUEST'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_SUCCESS = 'FETCH_PATIENT_CLINICAL_STATEMENTS_SUCCESS'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_FAILURE = 'FETCH_PATIENT_CLINICAL_STATEMENTS_FAILURE'; // Actions export const fetchPatientClinicalStatementsRequest = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_REQUEST); export const fetchPatientClinicalStatementsSuccess = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_SUCCESS); export const fetchPatientClinicalStatementsFailure = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_FAILURE); // Epics for async actions export const fetchPatientClinicalStatementsEpic = (action$, store) => {}; // reducer export default function reducer(patientsClinicalStatements = {}, action) { switch (action.type) { case FETCH_PATIENT_CLINICAL_STATEMENTS_SUCCESS: return _.set(action.payload.userId, action.payload.clinicalStatements, patientsClinicalStatements); default: return patientsClinicalStatements; } }
Clinical Statements Detail Duck
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; import { handleErrors } from '../../../../ducks/handle-errors.duck'; // Actions names export const FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_REQUEST = 'FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_REQUEST'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_SUCCESS = 'FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_SUCCESS'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_FAILURE = 'FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_FAILURE'; // Actions export const fetchPatientClinicalStatementsDetailRequest = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_REQUEST); export const fetchPatientClinicalStatementsDetailSuccess = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_SUCCESS); export const fetchPatientClinicalStatementsDetailFailure = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_FAILURE); // Epics for async actions export const fetchPatientClinicalStatementsDetailEpic = (action$, store) => {}; // reducer export default function reducer(clinicalStatementsDetail = {}, action) { switch (action.type) { case FETCH_PATIENT_CLINICAL_STATEMENTS_DETAIL_SUCCESS: return _.set(action.payload.userId, action.payload.clinicalStatementsDetail, clinicalStatementsDetail); default: return clinicalStatementsDetail; } }
Clinical Statements Create Duck
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; import { fetchPatientClinicalStatementsRequest } from './fetch-patient-clinical-statements.duck' import { handleErrors } from "../../../../ducks/handle-errors.duck"; // Actions names export const FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_REQUEST = 'FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_REQUEST'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_SUCCESS = 'FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_SUCCESS'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_FAILURE = 'FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_FAILURE'; // Actions export const fetchPatientClinicalStatementsCreateRequest = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_REQUEST); export const fetchPatientClinicalStatementsCreateSuccess = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_SUCCESS); export const fetchPatientClinicalStatementsCreateFailure = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_FAILURE); // Epics for async actions export const fetchPatientClinicalStatementsCreateEpic = (action$, store) => {}; // reducer export default function reducer(patientClinicalStatementsCreate = {}, action) { switch (action.type) { case FETCH_PATIENT_CLINICAL_STATEMENTS_CREATE_SUCCESS: return action.payload; default: return patientClinicalStatementsCreate } }
Clinical Statements Tags Duck
API URL
/api/contentStore/ts/tags
GET response
["acutesob", "chestpain", "earpain", "lung", "shoulder", "shoulder2", "snomedct", "t1", "tagb", …]
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; import { handleErrors } from '../../../../ducks/handle-errors.duck'; // Actions names export const FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_REQUEST = 'FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_REQUEST'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_SUCCESS = 'FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_SUCCESS'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_FAILURE = 'FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_FAILURE'; // Actions export const fetchPatientClinicalStatementsTagsRequest = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_REQUEST); export const fetchPatientClinicalStatementsTagsSuccess = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_SUCCESS); export const fetchPatientClinicalStatementsTagsFailure = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_FAILURE); // Epics for async actions export const fetchPatientClinicalStatementsTagsEpic = (action$, store) => {}; // reducer export default function reducer(patientsClinicalStatementsTags = {}, action) { switch (action.type) { case FETCH_PATIENT_CLINICAL_STATEMENTS_TAGS_SUCCESS: return _.set(action.payload.userId, action.payload.clinicalStatementsTags, patientsClinicalStatementsTags); default: return patientsClinicalStatementsTags; } }
Clinical Statements Query Duck
API URL
/api/contentStore/ts/phrases?tag={tag}
GET response
[ { id:7 phrase:"The pain was mild at |?| in severity" }, ... ]
File structure
// import packages import { Observable } from 'rxjs'; import { ajax } from 'rxjs/observable/dom/ajax'; import { createAction } from 'redux-actions'; import { handleErrors } from '../../../../ducks/handle-errors.duck'; // Actions names export const FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_REQUEST = 'FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_REQUEST'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_SUCCESS = 'FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_SUCCESS'; export const FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_FAILURE = 'FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_FAILURE'; // Actions export const fetchPatientClinicalStatementsQueryRequest = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_REQUEST); export const fetchPatientClinicalStatementsQuerySuccess = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_SUCCESS); export const fetchPatientClinicalStatementsQueryFailure = createAction(FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_FAILURE); // Epics for async actions export const fetchPatientClinicalStatementsQueryEpic = (action$, store) => {}; // reducer export default function reducer(patientsClinicalStatementsQuery = {}, action) { switch (action.type) { case FETCH_PATIENT_CLINICAL_STATEMENTS_QUERY_SUCCESS: return _.set(action.payload.tag, action.payload.clinicalStatementsQuery, patientsClinicalStatementsQuery); default: return patientsClinicalStatementsQuery; } }