Allergies module
General information
Allergies is a core plugin of PulseTile-RA. It is used to create, edit and review information about allergies of the current patient. Actions, Reducer and Sagas required for the Allergies plugin are created automatically by React-Admin framework, because all operations are typical.
Allergies
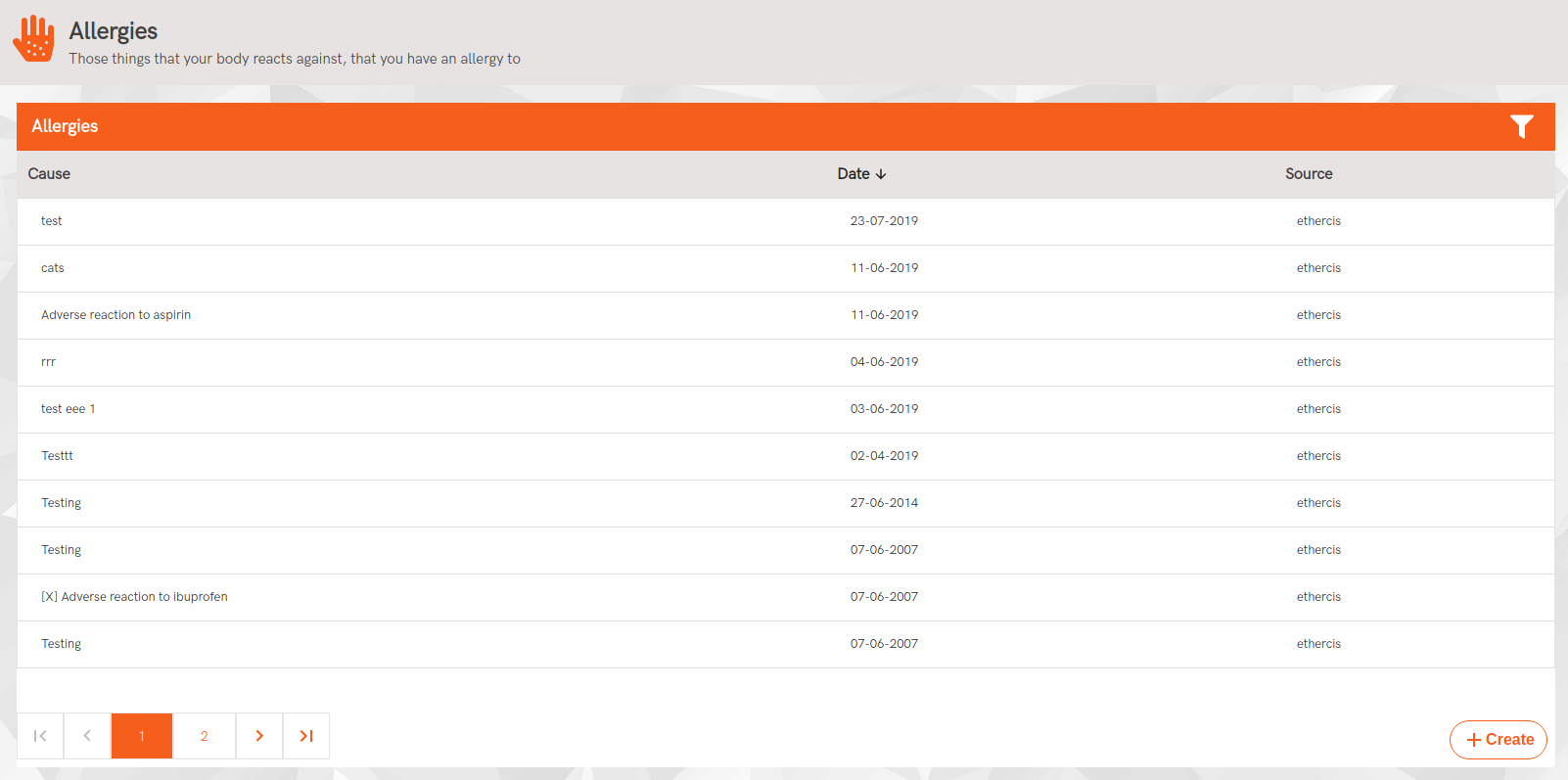
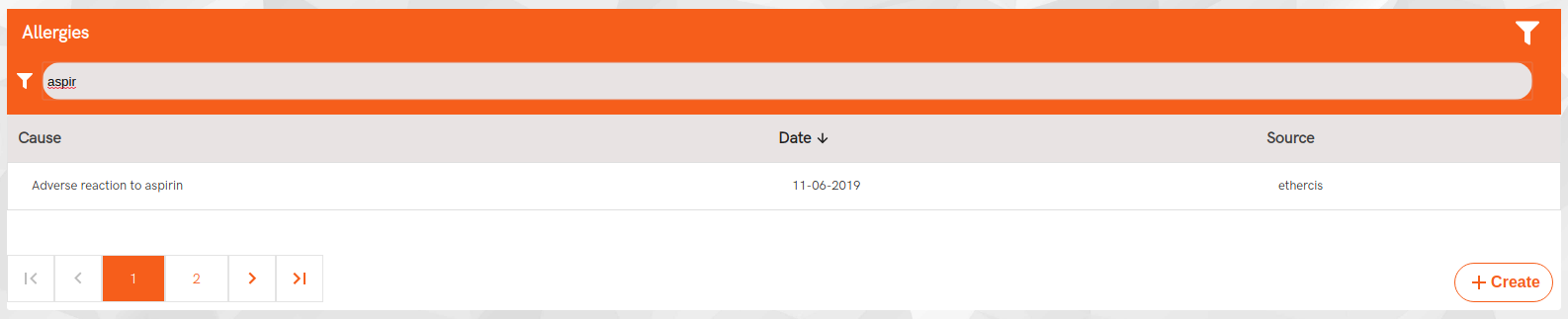
API URL
/api/patients/{patientId}/allergies
GET response
{ cause: "Adverse reaction to aspirin", dateCreated: 1181170800000, reaction: "Adverse reaction to aspirin", source: "ethercis", sourceId: "ethercis-11d2021c-f1a9-4258-800e-9e141b1521b4", }
Component structure
import React from "react"; import { TextField, DateField } from "react-admin"; import ListTemplate from "../../common/ResourseTemplates/ListTemplate"; import AllergiesCreate from "./AllergiesCreate"; import AllergiesEdit from "./AllergiesEdit"; import AllergiesShow from "./AllergiesShow"; import DatagridRow from "./fragments/DatagridRow"; const AllergiesList = props => ( <ListTemplate create={AllergiesCreate} edit={AllergiesEdit} show={AllergiesShow} resourceUrl="allergies" title="Allergies" CustomRow={DatagridRow} isCustomDatagrid={true} > <TextField source="cause" label="Cause" /> <DateField source="dateCreated" label="Date" /> <TextField source="source" label="Source" /> </ListTemplate> ); export default AllergiesList;
Allergies Detail
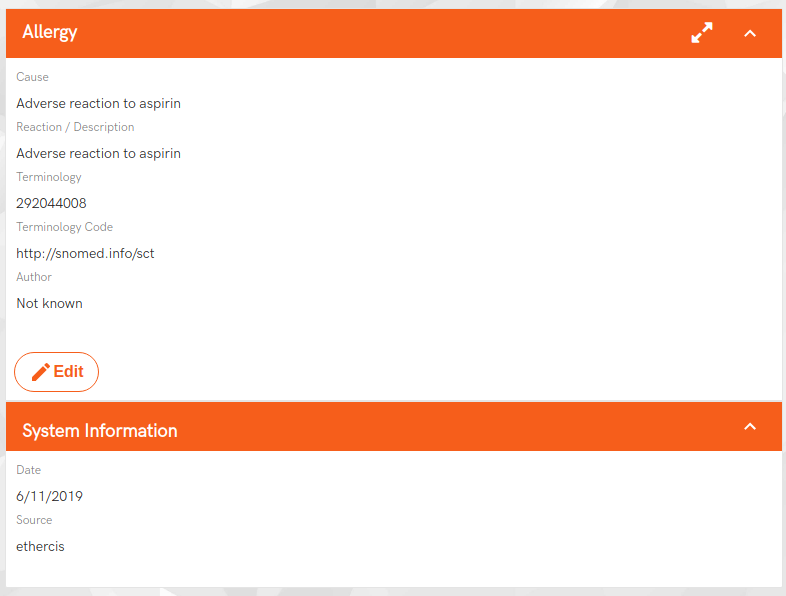
API URL
/api/patients/{patientId}/allergies/{sourceId}
GET response
{ author: "Not known", cause: "Adverse reaction to aspirin", causeCode: "http://snomed.info/sct", causeTerminology: 292044008, dateCreated: 1181170800000, originalComposition: "", originalSource: "", reaction: "Adverse reaction to aspirin", source: "ethercis", sourceId: "ethercis-11d2021c-f1a9-4258-800e-9e141b1521b4", terminologyCode: "http://snomed.info/sct", }
Component structure
import React from "react"; import { TextField, DateField } from "react-admin"; import ShowTemplate from "../../common/ResourseTemplates/ShowTemplate"; const AllergiesShow = ({ classes, ...rest }) => ( <ShowTemplate pageTitle="Allergy" {...rest}> <TextField label="Cause" source="cause" /> <TextField label="Reaction / Description" source="reaction" /> <TextField label="Author" source="author" /> <DateField label="Date" source="dateCreated" /> </ShowTemplate> ); export default AllergiesShow;
Allergies Edit Page
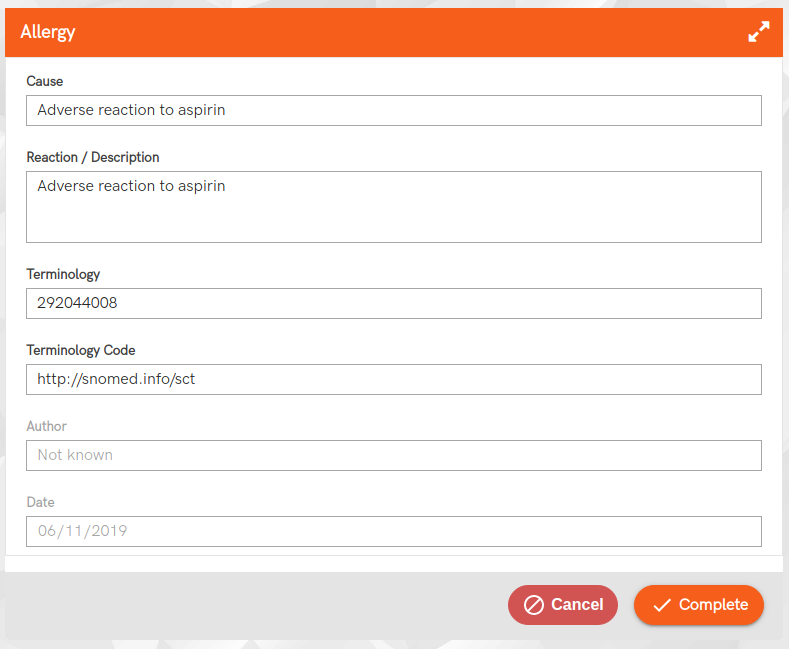
API URL
/api/patients/{patientId}/allergies/{sourceId}
PUT data
{ author: "Not known", cause: "Adverse reaction to aspirin", causeCode: "http://snomed.info/sct", causeTerminology: 292044008, dateCreated: 1181170800000, id: "ethercis-11d2021c-f1a9-4258-800e-9e141b1521b4", originalComposition: "", originalSource: "", reaction: "Adverse reaction to aspirin", source: "ethercis", sourceId: "ethercis-11d2021c-f1a9-4258-800e-9e141b1521b4", terminologyCode: "http://snomed.info/sct", userId: "9999999801", }
Component structure
import React from "react"; import EditTemplate from "../../common/ResourseTemplates/EditTemplate"; import Inputs from "./fragments/Inputs"; const AllergiesEdit = ({ classes, ...rest }) => ( <EditTemplate blockTitle="Allergy" {...rest}> <Inputs /> </EditTemplate> ); export default AllergiesEdit;
Allergies Create Page
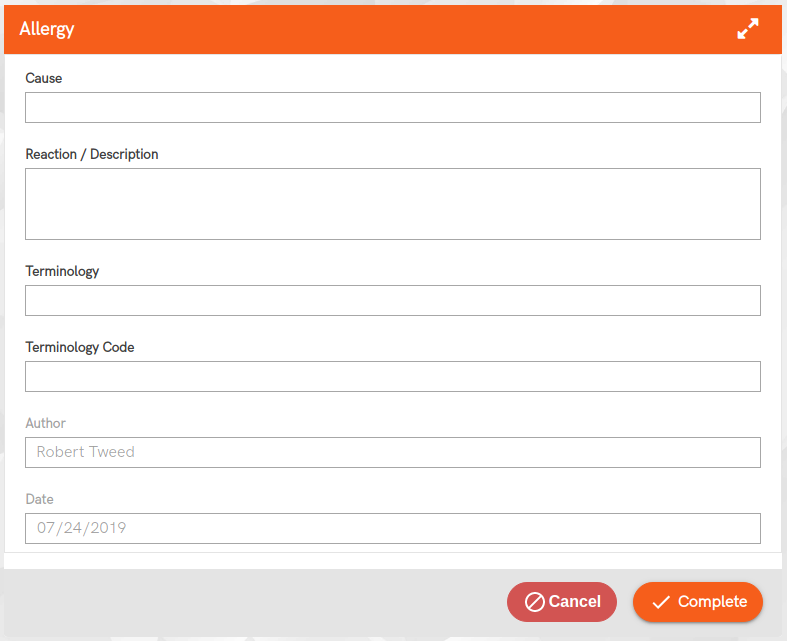
API URL
/api/patients/{patientId}/allergies
POST data
{ author: "Robert Tweed", cause: "cats", causeCode: "cats", causeTerminology: "cats", dateCreated: "06/11/2019", reaction: "cats", userId: "9999999801", }
Component structure
import React from "react"; import CreateTemplate from "../../common/ResourseTemplates/CreateTemplate"; import Inputs from "./fragments/Inputs"; const AllergiesCreate = props => ( <CreateTemplate blockTitle="Allergy" {...props}> <Inputs /> </CreateTemplate> ); export default AllergiesCreate;