Clinical Notes module
General information
Clinical Notes is a non-core plugin of PulseTile-RA. It is used to create, edit and review information about Clinical Notes of the current patient. Actions, Reducer and Sagas required for the Clinical Notes plugin are created automatically by React-Admin framework, because all operations are typical.
Clinical Notes List
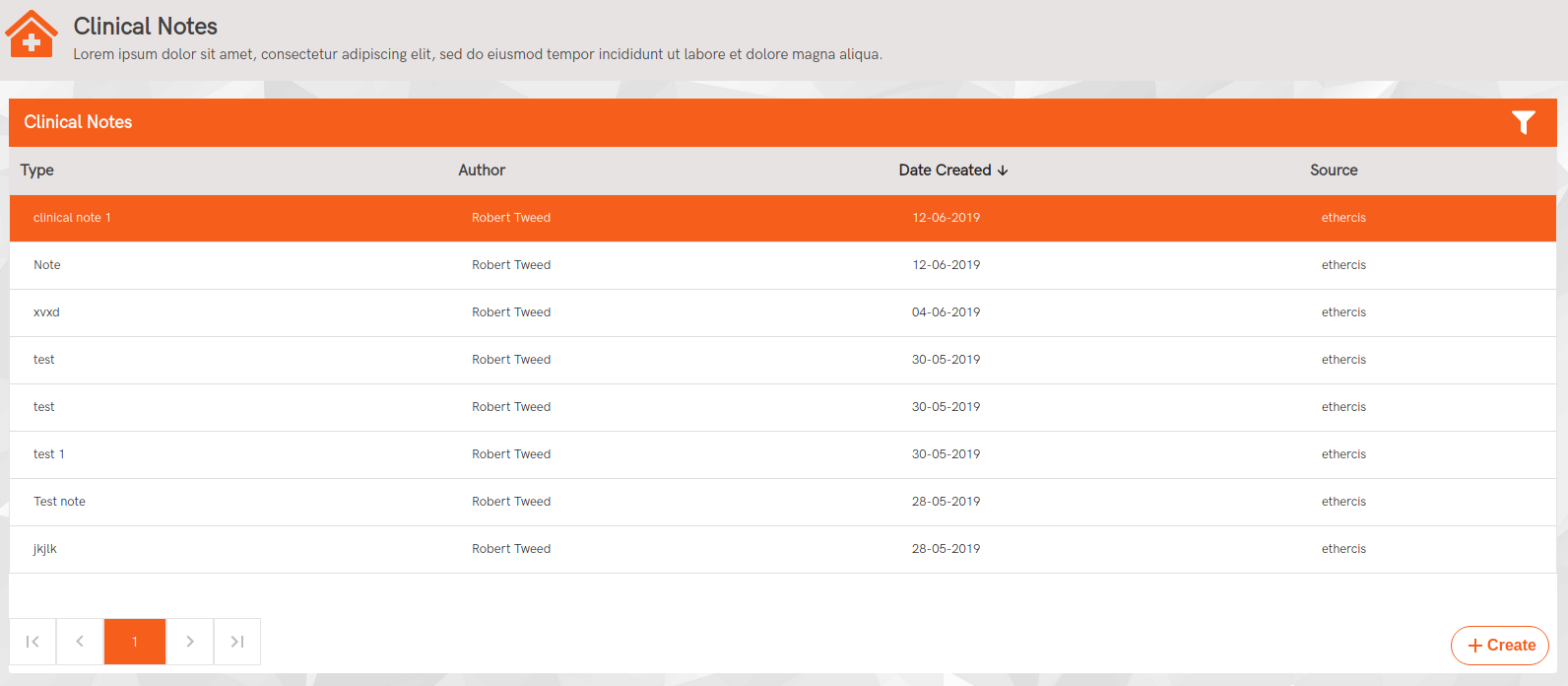
API URL
/api/patients/{patientId}/clinicalnotes
GET response
{ author: "Robert Tweed", clinicalNotesType: "test", dateCreated: 1559222911000, source: "ethercis", sourceId: "ethercis-5313f3a9-1038-44de-a2e1-453b3a34822c", }
Component structure
import React from "react"; import { DateField, TextField } from "react-admin"; import ListTemplate from "../../../core/common/ResourseTemplates/ListTemplate"; import ClinicalNotesCreate from "./ClinicalNotesCreate"; import ClinicalNotesEdit from "./ClinicalNotesEdit"; import ClinicalNotesShow from "./ClinicalNotesShow"; import DatagridRow from "./fragments/DatagridRow"; const PersonalNotesList = ({ classes, ...rest }) => ( <ListTemplate create={ClinicalNotesCreate} edit={ClinicalNotesEdit} show={ClinicalNotesShow} resourceUrl="clinicalnotes" title="Clinical Notes" CustomRow={DatagridRow} isCustomDatagrid={true} {...rest} > <TextField label="Type" source="clinicalNotesType" /> <TextField label="Author" source="author" /> <DateField label="Date Created" source="dateCreated" /> <TextField label="Source" source="source" /> </ListTemplate> ); export default PersonalNotesList;
Clinical Notes Detail
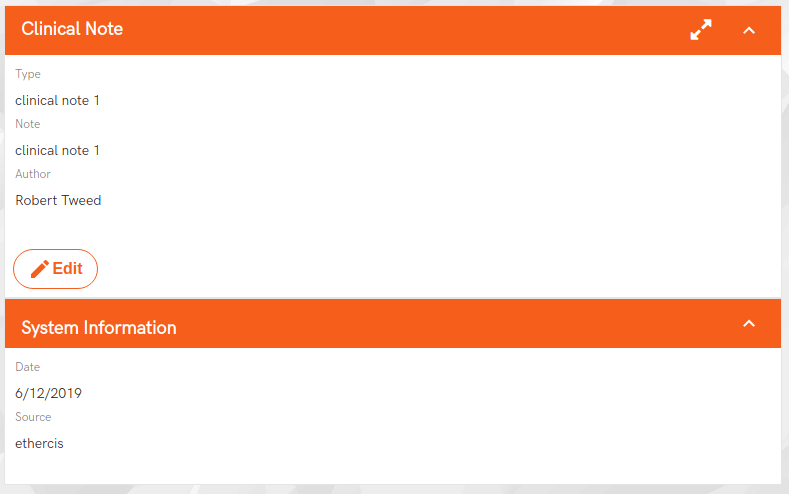
API URL
/api/patients/{patientId}/clinicalnotes/{sourceId}
GET response
{ author: "Robert Tweed", clinicalNotesType: "Note", dateCreated: 1559562808000, note: "test note", source: "ethercis", sourceId: "ethercis-2fd22d3b-8a5c-4563-ac6f-3f563b54179a", }
Component structure
import React from "react"; import { TextField, DateField } from "react-admin"; import { withStyles } from '@material-ui/core/styles'; import ShowTemplate from "../../../core/common/ResourseTemplates/ShowTemplate"; const PersonalNotesShow = ({ classes, ...rest }) => ( <ShowTemplate pageTitle="Clinical Note" {...rest}> <TextField className={classes.labelBlock} source="clinicalNotesType" label="Type" /> <TextField className={classes.labelBlock} source="note" label="Note" /> <TextField className={classes.labelBlock} source="author" label="Author" /> </ShowTemplate> ); export default withStyles(styles)(PersonalNotesShow);
Clinical Notes Edit Page
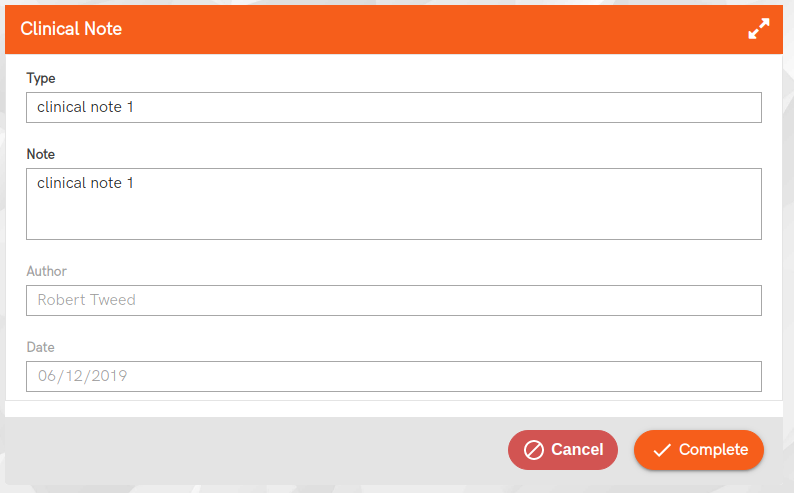
API URL
/api/patients/{patientId}/clinicalnotes/{sourceId}
PUT data
{ author: "Robert Tweed", clinicalNotesType: "Note", dateCreated: 1559562808000, id: "ethercis-2fd22d3b-8a5c-4563-ac6f-3f563b54179a", note: "test note", source: "ethercis", sourceId: "ethercis-2fd22d3b-8a5c-4563-ac6f-3f563b54179a", userId: "9999999801", }
Component structure
import React from "react"; import EditTemplate from "../../../core/common/ResourseTemplates/EditTemplate"; import Inputs from "./fragments/Inputs"; const ClinicalNotesEdit = ({ classes, ...rest }) => ( <EditTemplate blockTitle="Clinical Note" {...rest}> <Inputs /> </EditTemplate> ); export default ClinicalNotesEdit;
Clinical Notes Create Page
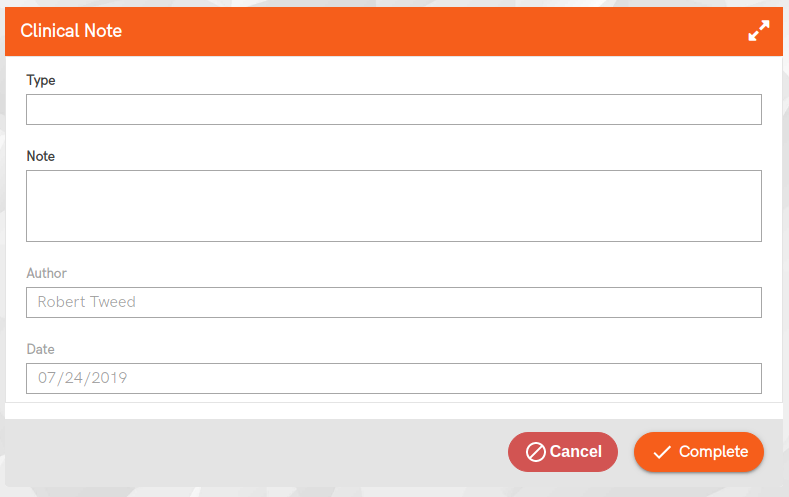
API URL
/api/patients/{patientId}/clinicalnotes
POST data
{ author: "Robert Tweed", clinicalNotesType: "clinical note 1", dateCreated: "06/12/2019", note: "clinical note 1", userId: "9999999801", }
Component structure
import React from "react"; import CreateTemplate from "../../../core/common/ResourseTemplates/CreateTemplate"; import Inputs from "./fragments/Inputs"; const ClinicalNotesCreate = ({ classes, ...rest }) => ( <CreateTemplate blockTitle="Clinical Note" {...rest}> <Inputs /> </CreateTemplate> ); export default ClinicalNotesCreate;