Medications module
General information
Medications is a core plugin of PulseTile-RA. It is used to create, edit and review information about medications of the current patient. Actions, Reducer and Sagas required for the Medications plugin are created automatically by React-Admin framework, because all operations are typical.
Medications
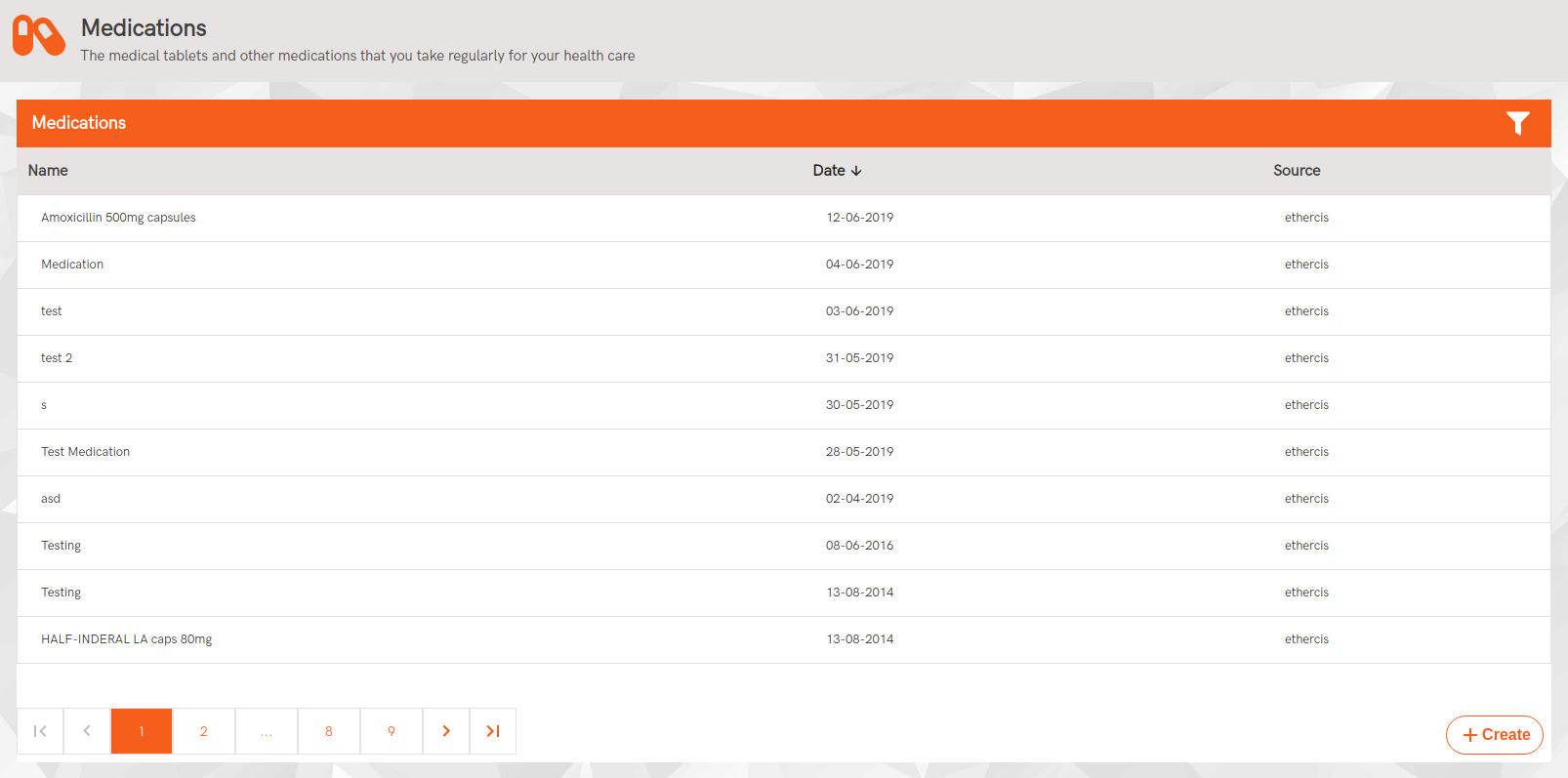
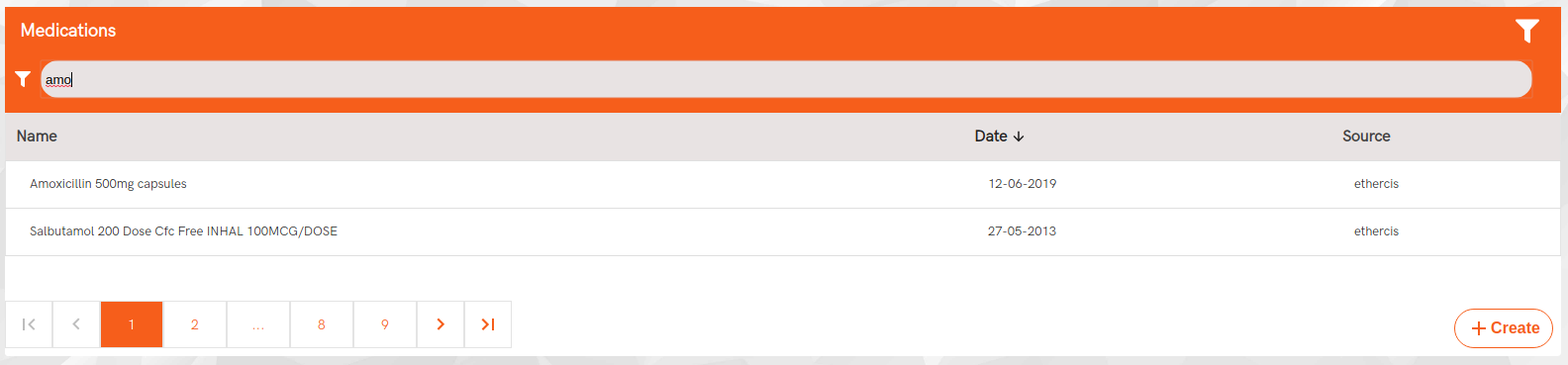
General information
Medications is a core plugin of PulseTile-RA. It is used to create, edit and review information about medications of the current patient. Actions, Reducer and Sagas required for the Medications plugin are created automatically by React-Admin framework, because all operations are typical.
API URL
/api/patients/{patientId}/medications
GET data
{ dateCreated: 1204243200000, doseAmount: "20mg", name: "Amoxicillin 500mg capsules", source: "ethercis", sourceId: "ethercis-5ad698d2-5c63-4da9-a4e4-53a017226699", }
Component structure
import React from "react"; import { DateField, TextField } from "react-admin"; import ListTemplate from "../../common/ResourseTemplates/ListTemplate"; import MedicationsCreate from "./MedicationsCreate"; import MedicationsEdit from "./MedicationsEdit"; import MedicationsShow from "./MedicationsShow"; import DatagridRow from "./fragments/DatagridRow"; export const MedicationsList = ({ classes, ...rest }) => ( <ListTemplate create={MedicationsCreate} edit={MedicationsEdit} show={MedicationsShow} resourceUrl="medications" title="Medications" CustomRow={DatagridRow} isCustomDatagrid={true} {...rest} > <TextField source="name" label="Name" /> <DateField source="dateCreated" label="Date" /> <TextField source="source" label="Source" /> </ListTemplate> ); export default MedicationsList;
Medications Detail
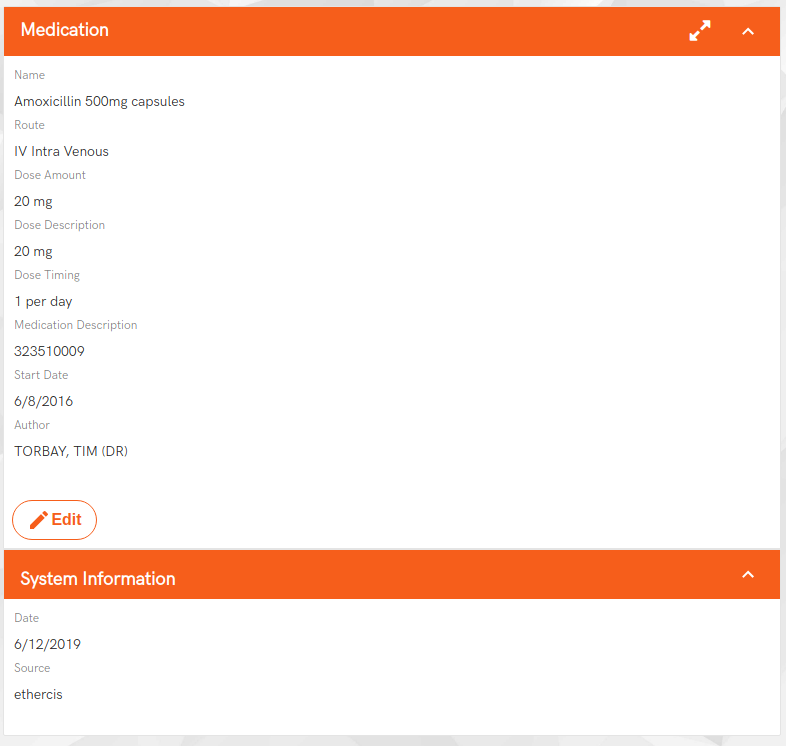
API URL
/api/patients/{patientId}/medications/{sourceId}
GET data
{ author: "TORBAY, TIM (DR)", dateCreated: 1465340400000, doseAmount: "", doseDirections: "", doseTiming: "Not known", medicationCode: 323510009, medicationTerminology: "http://snomed.info/sct", name: "Amoxicillin 500mg capsules", route: "Not known", source: "ethercis", sourceId: "ethercis-f28f0c62-04f4-434c-8f80-f3e7cdcbd425", startDate: 1465340400000, startTime: 0, }
Component structure
import React from "react"; import { TextField, DateField } from "react-admin"; import { withStyles } from '@material-ui/core/styles'; import ShowTemplate from "../../common/ResourseTemplates/ShowTemplate"; const ProblemsShow = ({ classes, ...rest }) => ( <ShowTemplate pageTitle="Medication" {...rest}> <TextField className={classes.labelBlock} label="Name" source="name" /> <TextField className={classes.labelBlock} label="Dose Description" source="doseAmount" /> <TextField className={classes.labelBlock} label="Author" source="author" /> <DateField className={classes.labelBlock} label="Date" source="dateCreated" /> </ShowTemplate> ); export default withStyles(styles)(ProblemsShow);
Medications Edit Page
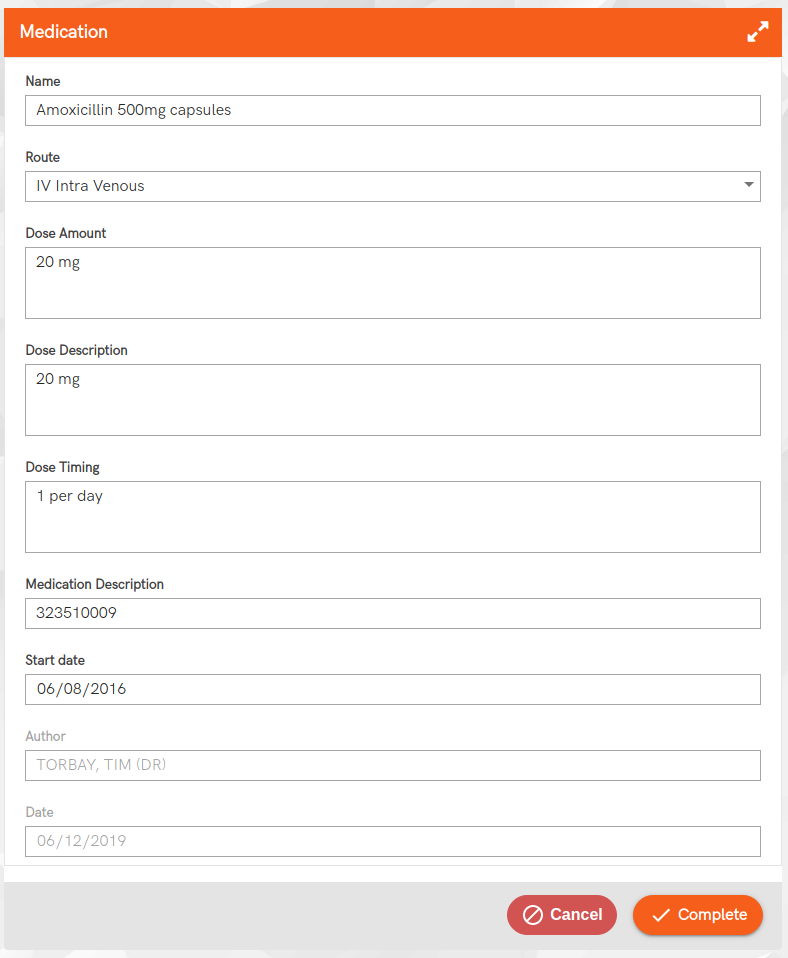
API URL
/api/patients/{patientId}/medications/{sourceId}
PUT data
{ author: "TORBAY, TIM (DR)", dateCreated: 1465340400000, doseAmount: "20 mg", doseDirections: "20 mg", doseTiming: "1 per day", id: "ethercis-f28f0c62-04f4-434c-8f80-f3e7cdcbd425", medicationCode: 323510009, medicationTerminology: "http://snomed.info/sct", name: "Amoxicillin 500mg capsules", route: "IV Intra Venous", source: "ethercis", sourceId: "ethercis-f28f0c62-04f4-434c-8f80-f3e7cdcbd425", startDate: 1465340400000, startTime: 0, userId: "9999999801", }
Component structure
import React from "react"; import EditTemplate from "../../common/ResourseTemplates/EditTemplate"; import Inputs from "./fragments/Inputs"; const MedicationsEdit = ({ classes, ...rest }) => ( <EditTemplate blockTitle="Medication" {...rest}> <Inputs /> </EditTemplate> ); export default MedicationsEdit;
Medications Create Page
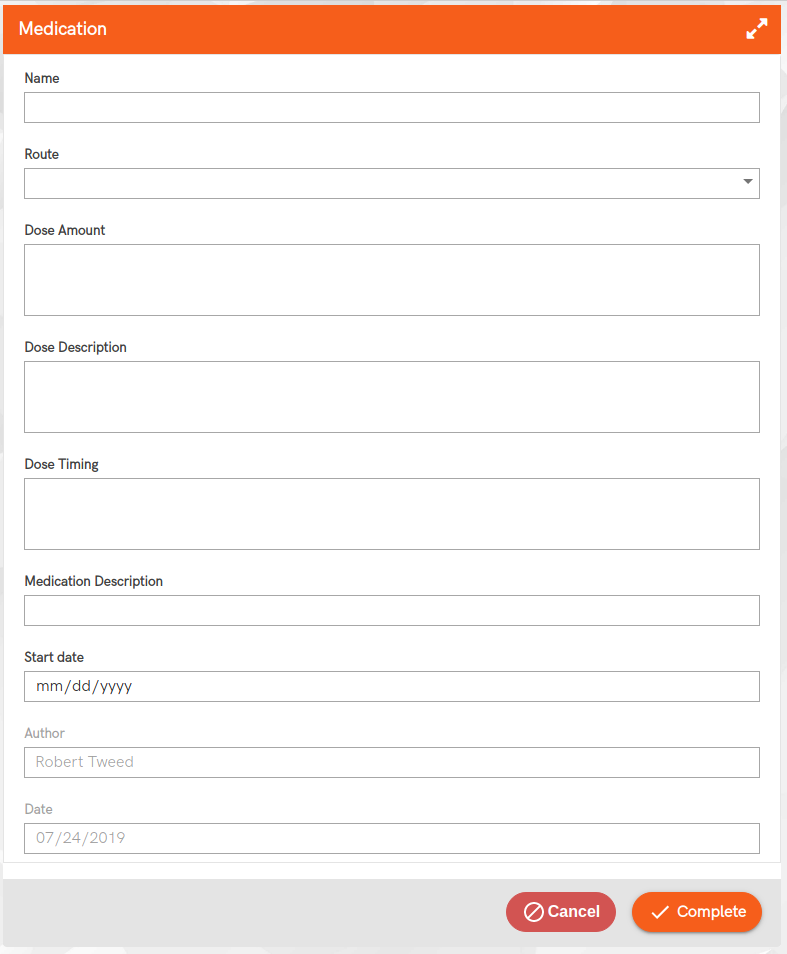
API URL
/api/patients/{patientId}/medications/{sourceId}
POST data
{ author: "Robert Tweed", dateCreated: "06/12/2019", doseAmount: "20 mg", doseDirections: "20 mg", doseTiming: "1 per day", medicationCode: "test", name: "Paracetomol", route: "Po Per Oral", startDate: "2019-06-12", startTime: "2019-06-15", userId: "9999999801", }
Component structure
import React from "react"; import CreateTemplate from "../../common/ResourseTemplates/CreateTemplate"; import Inputs from "./fragments/Inputs"; const MedicationsCreate = ({ classes, ...rest }) => ( <CreateTemplate blockTitle="Medication" {...rest}> <Inputs /> </CreateTemplate> ); export default MedicationsCreate;